在编程中,removeall
通常用于从集合或列表中移除所有匹配特定条件的元素,在使用这个方法时,可能会遇到各种错误,本文将详细探讨这些可能的错误及其解决方案,并提供一个常见问题解答(FAQs)部分。
常见错误及解决方案
1、TypeError: list indices must be integers or slices, not str
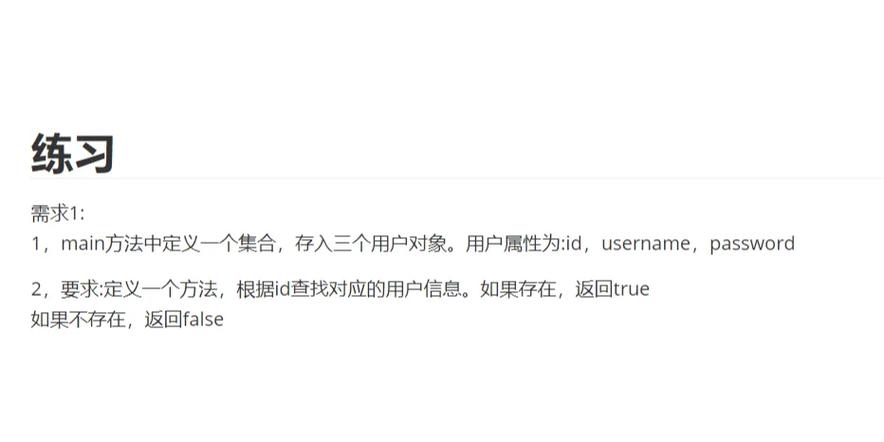
原因:尝试使用字符串索引列表。
解决方案:确保索引是整数或切片。
2、ValueError: list mutable, thus it cannot be hashed
原因:尝试将可变对象(如列表)用作字典键。
解决方案:使用不可变对象(如元组)作为字典键。
3、IndexError: list index out of range
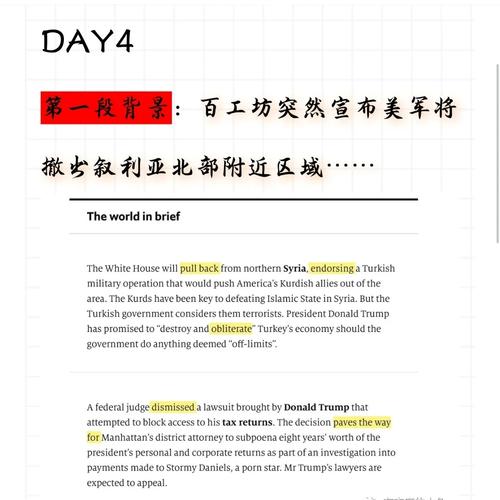
原因:尝试访问超出列表范围的索引。
解决方案:检查索引是否在有效范围内。
4、AttributeError: 'list' object has no attribute 'removeall'
原因:Python 标准库中没有removeall
方法。
解决方案:使用循环和remove
方法来替代。
5、TypeError: unhashable type: 'list'
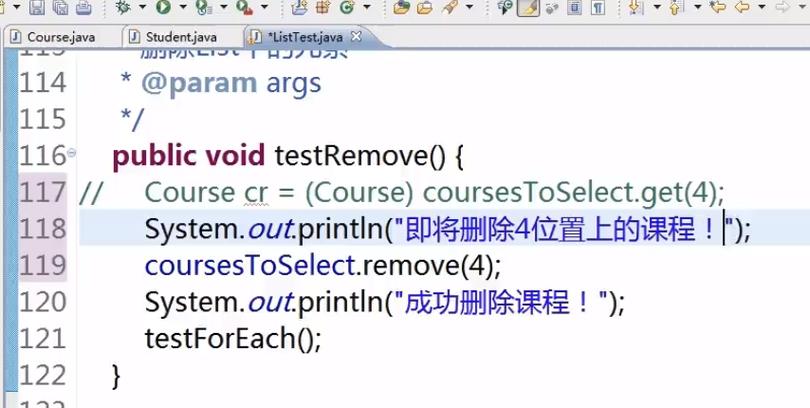
原因:尝试将列表用作字典键。
解决方案:使用元组或其他不可变类型作为字典键。
6、SyntaxError: invalid syntax
原因:语法错误,例如缺少冒号或括号不匹配。
解决方案:检查代码语法是否正确。
7、IndentationError: unexpected indent
原因:缩进不一致或不正确。
解决方案:确保所有代码块的缩进一致。
8、NameError: name 'removeall' is not defined
原因:未定义名为removeall
的函数或方法。
解决方案:定义所需的函数或使用正确的方法名。
9、RecursionError: maximum recursion depth exceeded
原因:递归调用过深。
解决方案:优化递归算法或增加递归深度限制。
10、MemoryError: out of memory
原因:程序消耗过多内存。
解决方案:优化内存使用或增加系统内存。
示例代码
以下是一些示例代码,演示如何使用不同的方法来移除列表中的所有匹配元素。
使用循环和remove
方法
- my_list = [1, 2, 3, 4, 3, 5]
- value_to_remove = 3
- while value_to_remove in my_list:
- my_list.remove(value_to_remove)
- print(my_list) # 输出: [1, 2, 4, 5]
使用列表推导式
- my_list = [1, 2, 3, 4, 3, 5]
- value_to_remove = 3
- my_list = [x for x in my_list if x != value_to_remove]
- print(my_list) # 输出: [1, 2, 4, 5]
使用filter
函数
- my_list = [1, 2, 3, 4, 3, 5]
- value_to_remove = 3
- my_list = list(filter(lambda x: x != value_to_remove, my_list))
- print(my_list) # 输出: [1, 2, 4, 5]
相关问答 FAQs
Q1: Python 中如何移除列表中的所有重复元素?
A1: 你可以使用集合来移除重复元素,然后再转换回列表。
- my_list = [1, 2, 2, 3, 4, 4, 5]
- unique_list = list(set(my_list))
- print(unique_list) # 输出可能是: [1, 2, 3, 4, 5](顺序可能不同)
Q2: Python 中如何移除列表中的所有 None 值?
A2: 你可以使用列表推导式来过滤掉所有的None
值。
- my_list = [1, None, 2, None, 3, None]
- cleaned_list = [x for x in my_list if x is not None]
- print(cleaned_list) # 输出: [1, 2, 3]
通过以上方法和建议,你可以有效地解决在使用removeall
时遇到的各种错误,并提高你的编程效率。