解决Spring Boot中的RestTemplate报错问题
在使用Spring Boot开发过程中,RestTemplate
是一个常用的HTTP客户端工具,开发者可能会遇到各种问题和错误,本文将详细探讨常见的RestTemplate
错误及其解决方法,并提供一些常见问题的解答。
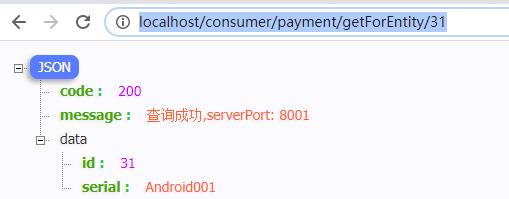
1. RestTemplate常见错误及解决方法
1.1 404 Not Found
描述:
请求的资源不存在或URL不正确。
解决方法:
确保请求的URL正确无误。
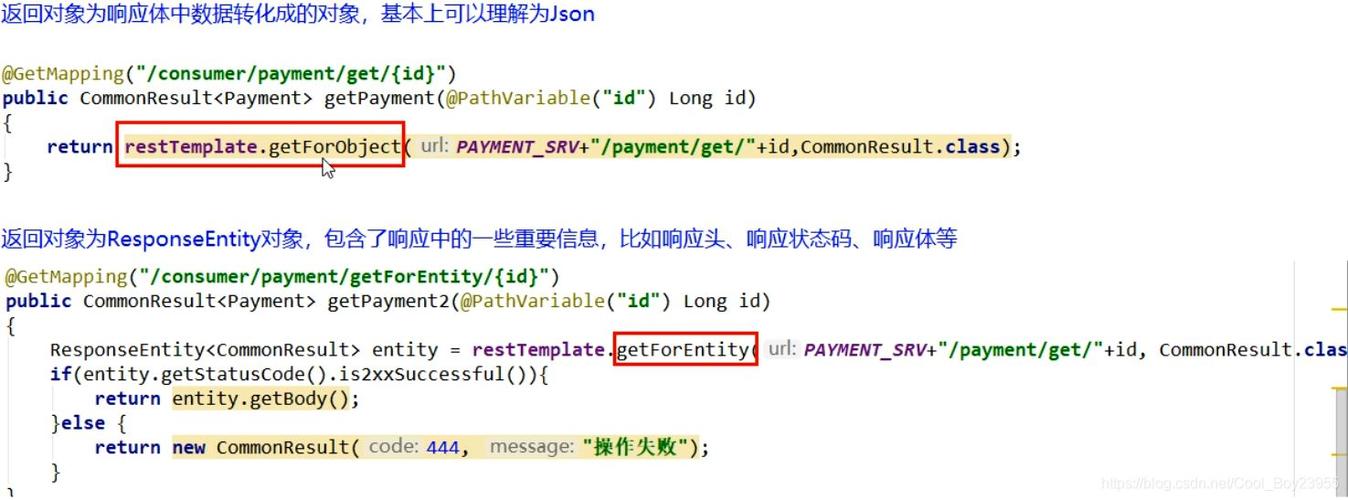
检查服务器端是否部署了相应的资源或API。
查看是否有权限访问该资源。
1.2 401 Unauthorized
描述:
请求未授权,通常是缺少认证信息。
解决方法:
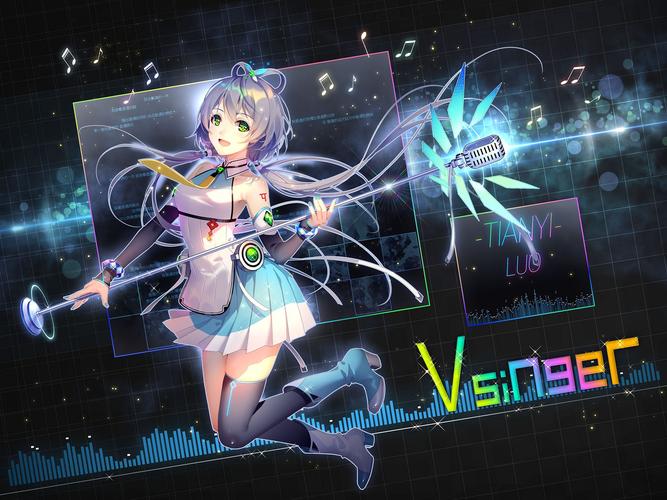
在请求头中添加认证信息,如Bearer Token或Basic Auth。
使用RestTemplate
的拦截器来自动添加认证信息。
1.3 403 Forbidden
描述:
服务器拒绝了请求,通常是由于权限不足。
解决方法:
确认用户有足够的权限访问资源。
检查服务器端的权限配置。
1.4 500 Internal Server Error
描述:
服务器内部错误,通常是服务器端的问题。
解决方法:
联系服务器管理员或开发人员,了解具体的错误原因。
检查服务器日志以获取更详细的错误信息。
1.5 超时错误
描述:
请求超时,可能是网络问题或服务器响应时间过长。
解决方法:
增加RestTemplate
的超时设置。
优化服务器性能,减少响应时间。
1.6 解析错误
描述:
无法解析响应数据,通常是JSON格式不正确。
解决方法:
确保服务器返回的数据是有效的JSON格式。
使用适当的数据类型进行解析。
代码示例
以下是一个简单的RestTemplate
使用示例:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.*; import org.springframework.stereotype.Service; import org.springframework.web.client.RestTemplate; import org.springframework.web.util.UriComponentsBuilder; @Service public class MyService { @Autowired private RestTemplate restTemplate; public String getData() { String url = "https://api.example.com/data"; HttpHeaders headers = new HttpHeaders(); headers.set("Authorization", "Bearer your_token"); HttpEntity<String> entity = new HttpEntity<>(headers); ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, entity, String.class); return response.getBody(); } }
FAQs
Q1: 如何为RestTemplate
设置超时?
A1: 你可以通过配置RequestFactory
来设置超时,以下是一个示例:
import org.springframework.boot.web.client.RestTemplateBuilder; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.http.client.ClientHttpRequestFactory; import org.springframework.http.client.SimpleClientHttpRequestFactory; @Configuration public class AppConfig { @Bean public RestTemplate restTemplate(RestTemplateBuilder builder) { return builder .requestFactory(() > new SimpleClientHttpRequestFactory()) .build(); } }
Q2: 如何处理RestTemplate
的异常?
A2: 你可以使用trycatch
块来捕获并处理异常,以下是一个示例:
try { ResponseEntity<String> response = restTemplate.getForEntity("https://api.example.com/data", String.class); System.out.println(response.getBody()); } catch (Exception e) { e.printStackTrace(); // 根据异常类型进行处理,例如重试、记录日志等 }
通过以上内容,你应该能够更好地理解和解决RestTemplate
在使用过程中遇到的各种问题,希望这些信息对你有所帮助!