BeautifulSoup 是一个用于从HTML和XML文件中提取数据的Python库,在使用 BeautifulSoup 时,可能会遇到各种报错,本文将详细介绍常见的错误类型及其解决方法,并提供相关FAQs以供参考。
常见错误及解决方案

1. 导入错误
错误信息:
ModuleNotFoundError: No module named 'beautifulsoup4'
原因分析:
这个错误通常是因为beautifulsoup4
模块没有被安装。
解决方案:
使用以下命令安装beautifulsoup4
和lxml
(一个解析器):
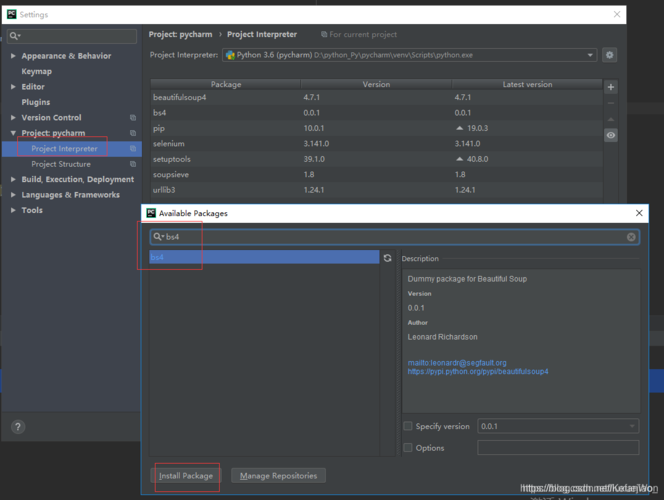
pip install beautifulsoup4 lxml
2. HTML解析错误
错误信息:
bs4.FeatureNotFound: Couldn't find a tree builder with the features you requested: lxml, html. Do you need to install a parser library?
原因分析:
这个错误表明在解析HTML时,没有找到合适的解析器,可能的原因是没有安装或正确配置解析器。
解决方案:
确保安装了lxml
或其他解析器,如果已经安装,请检查是否正确导入。
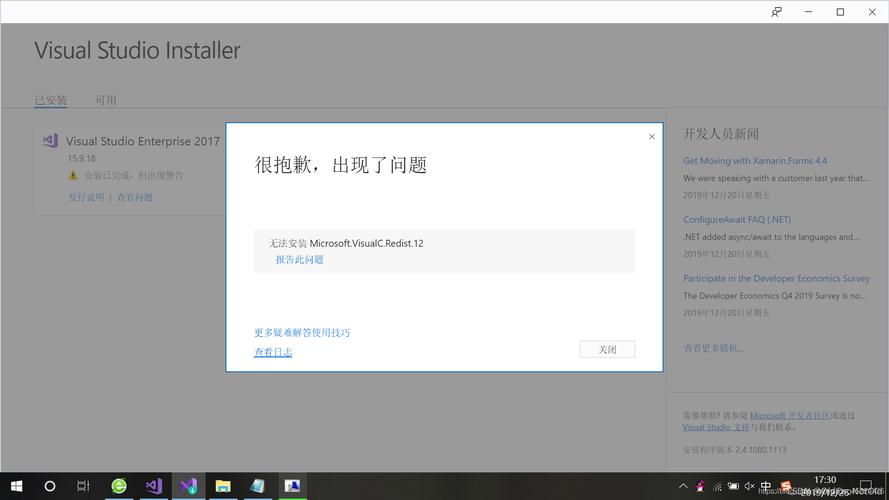
from bs4 import BeautifulSoup soup = BeautifulSoup(html_doc, 'lxml')
3. 标签选择错误
错误信息:
TypeError: 'NoneType' object is not subscriptable
原因分析:
这个错误通常发生在尝试访问不存在的标签或属性时。
解决方案:
确保选择的标签存在,并使用正确的语法。
错误的用法 title = soup.title[0] 正确的用法 title = soup.title.string if soup.title else None
4. 编码错误
错误信息:
UnicodeDecodeError: 'utf8' codec can't decode byte 0x80 in position 1234: invalid start byte
原因分析:
这个错误通常发生在处理非UTF8编码的HTML文件时。
解决方案:
指定正确的编码格式。
with open('file.html', 'r', encoding='iso88591') as file: soup = BeautifulSoup(file, 'lxml')
表格归纳
错误类型 | 错误信息 | 原因分析 | 解决方案 |
导入错误 | ModuleNotFoundError: No module named 'beautifulsoup4' | 未安装beautifulsoup4 模块 | pip install beautifulsoup4 lxml |
HTML解析错误 | bs4.FeatureNotFound: Couldn't find a tree builder with the features you requested: lxml, html. | 缺少合适的解析器 | 确保安装并正确导入解析器,如lxml |
标签选择错误 | TypeError: 'NoneType' object is not subscriptable | 尝试访问不存在的标签或属性 | 确保选择的标签存在,并使用正确的语法 |
编码错误 | UnicodeDecodeError: 'utf8' codec can't decode byte 0x80 in position 1234: invalid start byte | 处理非UTF8编码的HTML文件 | 指定正确的编码格式,如encoding='iso88591' |
相关问答FAQs
Q1: 如何确保BeautifulSoup使用正确的解析器?
A1: 在创建BeautifulSoup
对象时,第二个参数指定要使用的解析器,可以使用lxml
、html.parser
或html5lib
,推荐使用lxml
,因为它速度较快且容错性好,示例如下:
from bs4 import BeautifulSoup soup = BeautifulSoup(html_doc, 'lxml')
Q2: 如何处理BeautifulSoup中的嵌套标签?
A2: 可以使用.find_all()
或.select()
方法来查找嵌套标签,要查找所有的<p>
标签内的链接,可以这样做:
paragraphs = soup.find_all('p') for paragraph in paragraphs: links = paragraph.find_all('a') for link in links: print(link.get('href'))
或者使用 CSS 选择器:
links = soup.select('p > a') for link in links: print(link.get('href'))