HttpClient报错分析与解决方案
在使用HttpClient进行HTTP请求时,可能会遇到各种类型的错误,这些错误通常涉及网络连接问题、服务器响应问题以及客户端配置问题等,本文将详细解析常见的HttpClient错误类型,并提供相应的解决方案。
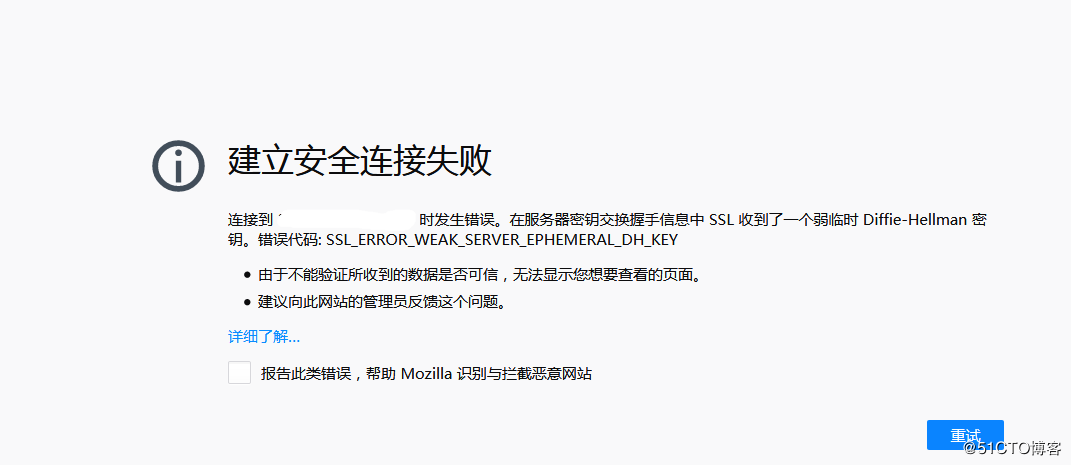
常见HttpClient错误类型及解决方案
一、java.net.ConnectException: Connection refused (Connection refused)
原因:服务端没有启动或客户端尝试连接的地址和端口不正确。
解决方案:
确保服务端已经启动并正在监听请求。
检查客户端请求的URL和端口是否正确。
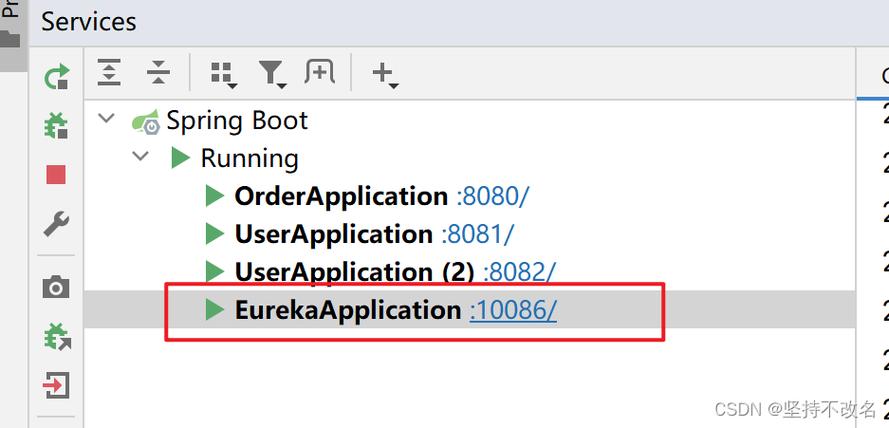
确认防火墙或安全组设置允许通过相应的端口。
示例代码:
- try {
- HttpGet request = new HttpGet("http://localhost:8080/test");
- CloseableHttpResponse response = httpClient.execute(request);
- } catch (ConnectException e) {
- System.out.println("服务端未启动或地址错误: " + e.getMessage());
- }
二、java.net.SocketTimeoutException: Read timed out
原因:服务端处理时间过长,导致客户端读取超时。
解决方案:
增加客户端的socket超时时间。
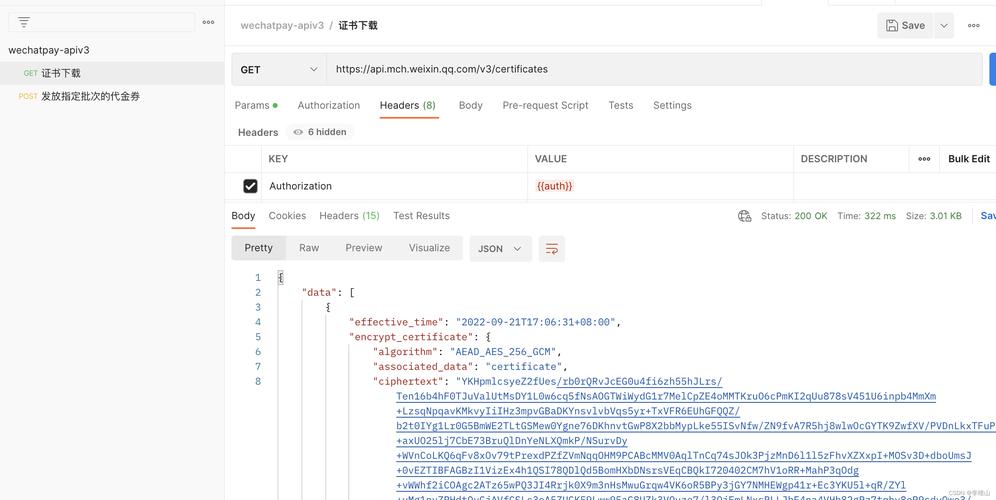
优化服务端的处理逻辑,提高响应速度。
示例代码:
- RequestConfig requestConfig = RequestConfig.custom()
- .setSocketTimeout(30000) // 设置读取超时时间为30秒
- .setConnectTimeout(10000) // 设置连接超时时间为10秒
- .build();
- HttpGet request = new HttpGet("http://localhost:8080/test");
- request.setConfig(requestConfig);
- try {
- CloseableHttpResponse response = httpClient.execute(request);
- } catch (SocketTimeoutException e) {
- System.out.println("请求超时: " + e.getMessage());
- }
三、org.apache.commons.httpclient.NoHttpResponseException
原因:服务端负载高,无法及时处理客户端的请求,导致连接被关闭。
解决方案:
建议客户端重试请求。
优化服务端性能,增加worker线程数或其他资源。
示例代码:
- HttpRequestRetryHandler myRetryHandler = new HttpRequestRetryHandler() {
- public boolean retryRequest(IOException exception, int executionCount, HttpContext context) {
- if (executionCount >= 5) {
- return false;
- }
- if (exception instanceof NoHttpResponseException) {
- return true;
- }
- return false;
- }
- };
- CloseableHttpClient httpClient = HttpClients.custom()
- .setRetryHandler(myRetryHandler)
- .build();
- try {
- HttpGet request = new HttpGet("http://localhost:8080/test");
- CloseableHttpResponse response = httpClient.execute(request);
- } catch (NoHttpResponseException e) {
- System.out.println("服务端无响应,重试请求: " + e.getMessage());
- }
四、java.net.SocketException: Connection reset by peer (connect failed)
原因:一端的Socket被关闭,而另一端仍在发送数据,服务器的并发连接数超过了其承载量,服务器会将其中一些连接关闭。
解决方案:
检查服务器的最大并发连接数设置,并进行优化。
在客户端捕获该异常并进行相应处理,如重试请求。
示例代码:
- try {
- HttpGet request = new HttpGet("http://localhost:8080/test");
- CloseableHttpResponse response = httpClient.execute(request);
- } catch (SocketException e) {
- System.out.println("连接被重置: " + e.getMessage());
- // 可以选择重试请求
- }
五、java.io.IOException: Broken pipe
原因:在连接被重置后继续写数据导致的异常。
解决方案:
捕获该异常并进行相应处理,如记录日志或重试请求。
示例代码:
- try {
- HttpGet request = new HttpGet("http://localhost:8080/test");
- CloseableHttpResponse response = httpClient.execute(request);
- } catch (IOException e) {
- System.out.println("管道破裂: " + e.getMessage());
- // 可以选择重试请求
- }
使用HttpClient进行HTTP请求时可能遇到的常见错误包括连接拒绝、读取超时、无HTTP响应、连接重置以及管道破裂等,针对不同的错误,可以通过调整客户端配置、优化服务端性能以及合理处理异常来解决问题,在实际开发中,建议结合具体的错误信息和上下文进行详细的排查和处理。