在开发过程中,AutoMapper 是一个非常有用的工具,用于在不同对象之间进行映射,有时在使用 AutoMapper 时会遇到各种报错问题,下面将详细探讨一些常见的 AutoMapper 报错原因及其解决方法:
1、字段名称不匹配:如果源对象和目标对象的字段名称不一致,需要手动配置映射规则,假设有一个源对象Source
和一个目标对象Destination
:
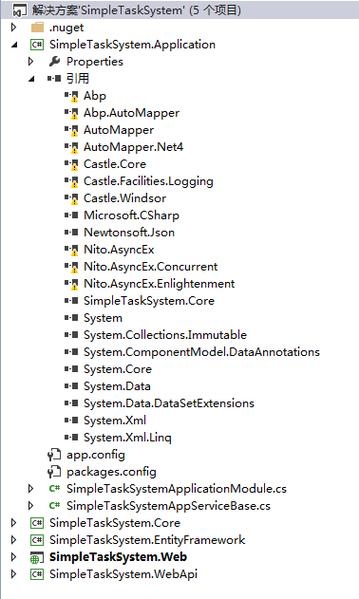
public class Source { public int Id { get; set; } public string Name { get; set; } } public class Destination { public int Identifier { get; set; } public string FullName { get; set; } }
在这种情况下,需要创建一个映射配置文件来定义如何将Source
映射到Destination
:
public class MappingProfile : Profile { public MappingProfile() { CreateMap<Source, Destination>() .ForMember(dest => dest.Identifier, opt => opt.MapFrom(src => src.Id)) .ForMember(dest => dest.FullName, opt => opt.MapFrom(src => src.Name)); } }
2、数据类型不匹配:当源对象和目标对象的数据类型不一致时,也需要手动配置映射规则,假设源对象中的某个字段是字符串类型,而目标对象中的对应字段是整数类型:
public class Source { public string AgeString { get; set; } } public class Destination { public int Age { get; set; } }
在这种情况下,可以这样配置映射:
public class MappingProfile : Profile { public MappingProfile() { CreateMap<Source, Destination>() .ForMember(dest => dest.Age, opt => opt.MapFrom(src => int.Parse(src.AgeString))); } }
3、集合映射问题:当需要映射包含集合的对象时,也需要注意,假设有一个源对象包含一个用户列表,需要将其映射到一个包含用户信息的 DTO 对象:
public class User { public string Name { get; set; } public int Age { get; set; } } public class UserDto { public string FullName { get; set; } public int Years { get; set; } } public class Source { public List<User> Users { get; set; } } public class Destination { public List<UserDto> UserDTOs { get; set; } }
在这种情况下,可以这样配置映射:
public class MappingProfile : Profile { public MappingProfile() { CreateMap<User, UserDto>() .ForMember(dest => dest.FullName, opt => opt.MapFrom(src => src.Name)) .ForMember(dest => dest.Years, opt => opt.MapFrom(src => src.Age)); CreateMap<Source, Destination>() .ForMember(dest => dest.UserDTOs, opt => opt.MapFrom(src => src.Users)); } }
4、反射异常:在某些情况下,使用反射将 DataRow 转换为实体类时可能会遇到问题,确保正确使用反射方法,并在转换前验证数据类型和结构。
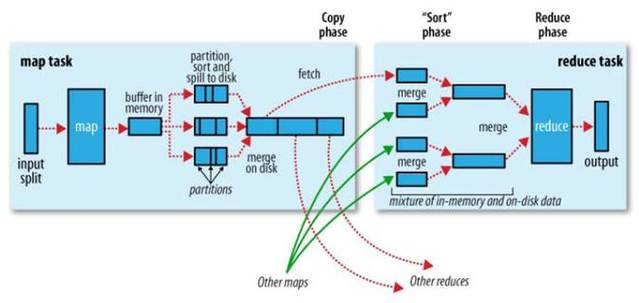
5、导航属性映射:当映射包含导航属性的实体时,也会遇到问题,假设有一个服务模型类User
和一个实体模型类UserData
:
public class User { public Guid UserId { get; set; } public string Name { get; set; } public Company Company { get; set; } } public class Company { public Guid CompanyId { get; set; } public string Name { get; set; } public ICollection<User> Users { get; set; } } public class UserData { public Guid Id { get; set; } public string Name { get; set; } public Guid CompanyId { get; set; } public virtual Company Company { get; set; } }
在这种情况下,需要正确配置映射关系:
public class UserProfile : Profile { public UserProfile() { CreateMap<UserData, User>() .ForMember(u => u.UserId, opt => opt.MapFrom(u => u.Id)) .ForMember(u => u.Company, opt => opt.MapFrom(u => u.Company)); } } public class CompanyProfile : Profile { public CompanyProfile() { CreateMap<CompanyData, Company>() .ForMember(o => o.CompanyId, opt => opt.MapFrom(o => o.Id)) .ForMember(o => o.Users, opt => opt.MapFrom(o => o.Users)); } }
6、静态方法配置问题:在使用 .NET Core 时,如果使用静态方法配置 AutoMapper,可能会遇到 “Mapper not initialized” 错误,确保通过依赖注入或适当的方式初始化 AutoMapper。
services.AddAutoMapper(typeof(Startup).Assembly); // Ensure all profiles are scanned and registered.
FAQs: AutoMapper 常见问题解答
1、Q1: AutoMapper 报“Missing type map configuration or unsupported mapping.”错误怎么办?
A1: 这个错误通常表示没有找到相应的映射配置,请确保已经创建了正确的映射配置文件,并且在启动时进行了扫描和注册,在 .NET Core 项目中,可以在Startup.cs
文件中添加以下代码:
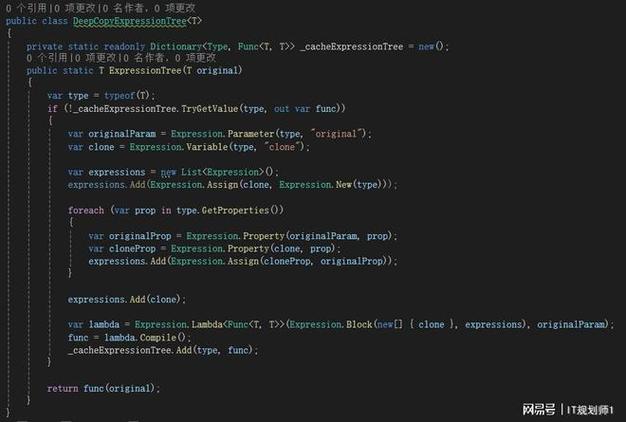
services.AddAutoMapper(typeof(MobanProfile).Assembly); // Ensure the profile is scanned and registered.
2、Q2: AutoMapper 报“Method 'Add' in type 'Proxy_System.Collections.Generic.ICollection`1...' from assembly 'AutoMapper.Proxies...' does not have an implementation.”错误怎么办?
A2: 这个错误通常与导航属性的映射有关,请检查映射配置是否正确,并确保源对象和目标对象的结构一致,对于包含集合的映射,确保正确配置了集合成员的映射关系,如:
CreateMap<Data.Entities.Company, Models.Company>() .ForMember(o => o.CompanyId, opt => opt.MapFrom(o => o.Id)) .ForMember(o => o.Users, opt => opt.MapFrom(o => o.Users));