报错null
解析与处理
在编程中,遇到null
错误是一个常见的问题。null
通常表示一个变量没有指向任何对象或值,这种错误可能会在各种编程语言中出现,Java、JavaScript、Python等,本文将详细解析null
错误的成因、常见场景、解决方法以及一些相关的FAQs。

一、null
错误的成因
1、未初始化的变量:在使用变量之前,如果没有对其进行初始化,可能会导致null
错误。
2、空指针引用:尝试访问或操作一个为null
的对象的属性或方法时,会引发null
错误。
3、数组越界:在访问数组元素时,如果索引超出数组范围,也可能导致null
错误。
4、数据库查询结果为空:从数据库查询数据时,如果查询结果为空,可能会导致null
错误。
5、API返回值为空:调用外部API时,如果返回值为空,也可能导致null
错误。

二、常见场景与解决方法
Java中的 `null` 错误
场景一:未初始化的变量
public class Main { public static void main(String[] args) { String str; System.out.println(str); // 这里会抛出 NullPointerException } }
解决方法:在使用变量之前进行初始化
public class Main { public static void main(String[] args) { String str = "Hello, World!"; System.out.println(str); } }
2. JavaScript中的null
错误
场景二:空指针引用
let obj = null; console.log(obj.property); // 这里会抛出 TypeError: Cannot read property 'property' of null
解决方法:在使用对象之前检查是否为null
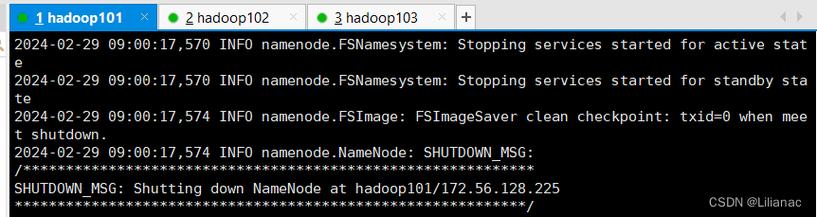
let obj = null; if (obj !== null) { console.log(obj.property); } else { console.log("Object is null"); }
3. Python中的null
错误
场景三:数组越界
arr = [1, 2, 3] print(arr[3]) # 这里会抛出 IndexError: list index out of range
解决方法:检查数组索引是否在范围内
arr = [1, 2, 3] if len(arr) > 3: print(arr[3]) else: print("Index out of range")
数据库查询结果为空
场景四:数据库查询结果为空
SELECT * FROM users WHERE id = 999; id=999 不存在,则结果为空
解决方法:检查查询结果是否为空
// 假设使用JDBC连接数据库 ResultSet rs = stmt.executeQuery("SELECT * FROM users WHERE id = 999"); if (rs.next()) { String name = rs.getString("name"); System.out.println(name); } else { System.out.println("No user found with the given ID"); }
API返回值为空
场景五:API返回值为空
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data.property)) // data 为 null,则会抛出错误
解决方法:检查API返回值是否为空
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { if (data !== null) { console.log(data.property); } else { console.log("Data is null"); } });
三、相关问答FAQs
Q1:什么是 `null`?
A1:null
是一个特殊的字面量,表示“没有值”或“空”,它用于表示变量尚未初始化或不指向任何对象,在很多编程语言中,null
是区分已分配内存但未赋值和未分配内存的一种方式。
Q2:如何避免 `null` 错误?
A2:避免null
错误的几种常见方法包括:
在使用变量之前进行初始化。
在使用对象之前检查是否为null
。
检查数组索引是否在范围内。
检查数据库查询结果是否为空。
检查API返回值是否为空。
通过这些方法,可以有效地减少null
错误的发生,提高代码的稳定性和可靠性。
理解和正确处理null
错误对于编写健壮的代码至关重要,通过上述方法和建议,开发者可以更好地应对null
错误,提高代码的质量和可维护性。