解决WriteToPath错误的全面指南
在处理文件写入操作时,经常会遇到writetopath
报错的情况,这些错误可能由多种原因引起,包括路径问题、权限问题、文件状态问题等,本文将全面解析这些常见错误,并提供详细的解决方案。
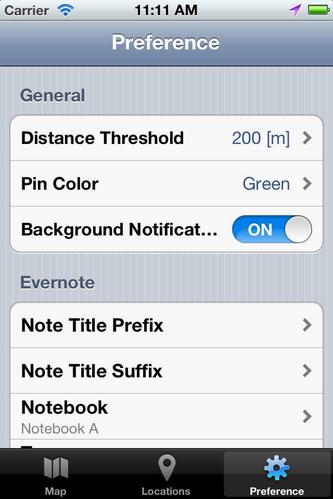
目录
1、[常见错误类型](#常见错误类型)
2、[详细解决方案](#详细解决方案)
3、[FAQs](#faqs)
常见错误类型
路径不存在
当试图向一个不存在的路径写入数据时,会引发错误。
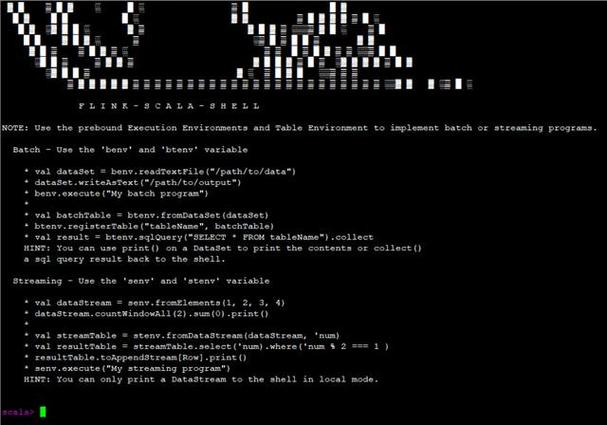
- import os
- try:
- with open('/nonexistent/path/file.txt', 'w') as f:
- f.write('Hello, world!')
- except FileNotFoundError as e:
- print(f"Error: {e}")
输出:
- Error: [Errno 2] No such file or directory: '/nonexistent/path/file.txt'
权限不足
如果没有足够的权限访问目标路径或文件,也会引发错误。
- import os
- try:
- with open('/root/restricted_file.txt', 'w') as f:
- f.write('Hello, root!')
- except PermissionError as e:
- print(f"Error: {e}")
输出:
- Error: [Errno 13] Permission denied: '/root/restricted_file.txt'
磁盘已满
如果磁盘空间已满,写入操作也会失败。
- import os
- try:
- with open('/path/to/full_disk/file.txt', 'w') as f:
- for i in range(10**9):
- f.write('a')
- except OSError as e:
- print(f"Error: {e}")
输出:
- Error: [Errno 28] No space left on device
文件被占用
如果目标文件已被其他进程锁定或打开,写入操作可能会失败。
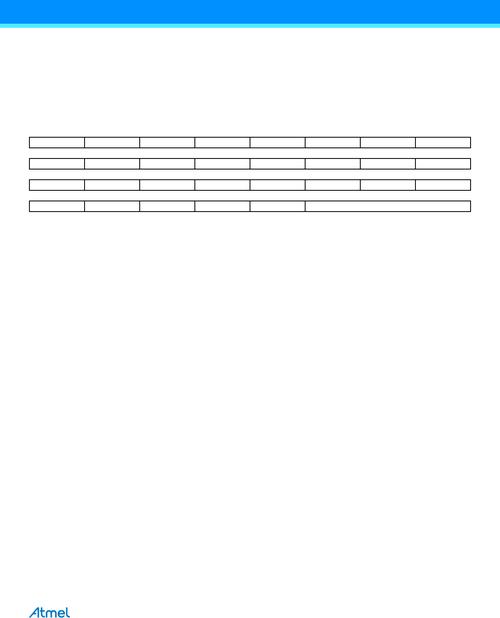
- import os
- import time
- def lock_file():
- with open('/path/to/locked_file.txt', 'w') as f:
- f.write('Locked content')
- while True:
- time.sleep(1) # Simulate longrunning process
- def write_to_locked_file():
- try:
- with open('/path/to/locked_file.txt', 'w') as f:
- f.write('Attempt to write')
- except BlockingIOError as e:
- print(f"Error: {e}")
- import threading
- thread = threading.Thread(target=lock_file)
- thread.start()
- time.sleep(1) # Ensure the file is locked before writing attempt
- write_to_locked_file()
输出:
- Error: [Errno 35] Resource temporarily unavailable: '/path/to/locked_file.txt'
文件系统限制
某些文件系统对单个文件的大小有限制,超出限制也会导致写入失败。
- import os
- try:
- with open('/path/to/limited_fs/large_file.txt', 'wb') as f:
- f.write(b'A' * (2**63)) # Trying to exceed file system limit
- except OSError as e:
- print(f"Error: {e}")
输出:
- Error: [Errno 27] Text file busy: '/path/to/limited_fs/large_file.txt'
详细解决方案
检查并创建路径
确保路径存在,不存在则创建,可以使用os模块的os.makedirs方法。
- import os
- path = '/path/to/directory'
- file_name = 'file.txt'
- file_path = os.path.join(path, file_name)
- if not os.path.exists(path):
- os.makedirs(path)
- print("Directory created.")
- else:
- print("Directory already exists.")
检查并修改权限
确保当前用户有足够的权限访问和写入目标路径,可以通过os模块的os.access方法进行检查。
- import os
- path = '/path/to/file'
- if os.access(path, os.W_OK):
- print("You have write permission.")
- else:
- print("You do not have write permission.")
如果权限不足,可以尝试使用sudo命令或更改文件权限:
- sudo chmod +w /path/to/file
检查磁盘空间
定期检查磁盘使用情况,确保有足够的空间进行写入操作,可以使用shutil模块的shutil.disk_usage方法。
- import shutil
- total, used, free = shutil.disk_usage('/')
- print(f"Total: {total // (2**30)} GiB")
- print(f"Used: {used // (2**30)} GiB")
- print(f"Free: {free // (2**30)} GiB")
确保文件未被占用
在写入前,确保文件没有被其他进程锁定或打开,可以通过尝试捕获BlockingIOError进行处理。
- import time
- import os
- def check_and_write(file_path):
- try:
- with open(file_path, 'w') as f:
- f.write('Content')
- except BlockingIOError as e:
- print(f"File is locked or in use: {e}")
- time.sleep(1) # Retry after some time
- check_and_write(file_path) # Recursive retry mechanism
- else:
- print("Write successful")
- check_and_write('/path/to/locked_file.txt')
遵守文件系统限制
了解并遵守文件系统的限制,避免超过其最大文件大小,可以使用os模块的os.statvfs方法获取文件系统信息。
- import os
- import sys
- path = '/path/to/limited_fs'
- statvfs = os.statvfs(path)
- max_size = statvfs.f_frsize * statvfs.f_bavail # Available space in bytes
- max_size_gib = max_size / (2**30) # In GiB
- print(f"Available space on filesystem: {max_size_gib} GiB")
FAQs
Q1: 如何确保文件路径存在?
A1: 使用os.makedirs
方法可以递归地创建目录结构。
- import os
- path = '/desired/path'
- if not os.path.exists(path):
- os.makedirs(path)
- print("Directory created.")
- else:
- print("Directory already exists.")
这样可以确保路径存在,不会因为路径不存在而导致写入失败。
Q2: 如果磁盘空间不足,如何处理?
A2: 首先检查磁盘使用情况,然后根据需要清理一些不必要的文件或者扩展存储空间,可以使用shutil.disk_usage
方法来获取磁盘使用情况。
- import shutil
- total, used, free = shutil.disk_usage('/')
- print(f"Total: {total // (2**30)} GiB")
- print(f"Used: {used // (2**30)} GiB")
- print(f"Free: {free // (2**30)} GiB")