import java.util.InputMismatchException; import java.util.Scanner; public class NextIntErrorHandling { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int num = 0; while (true) { try { System.out.print("请输入一个非负正整数:"); num = scanner.nextInt(); if (num > 0) { break; } else { System.out.println("输入的数不是非负正整数,请重新输入。"); } } catch (InputMismatchException e) { System.out.println("输入异常:" + e.toString()); scanner.next(); // 读取并丢弃错误的输入 } } System.out.println("您输入的非负正整数是:" + num); System.out.println("这个数的阶乘是:" + factorial(num)); scanner.close(); } public static long factorial(int n) { if (n == 1) { return 1; } else { return n * factorial(n 1); } } }
解释与分析
在上述代码中,我们处理了nextInt()
方法可能出现的错误,确保程序能够正确处理用户输入并计算输入数字的阶乘,以下是详细解释:
1、初始化Scanner对象:创建一个Scanner
对象用于从控制台读取用户输入。
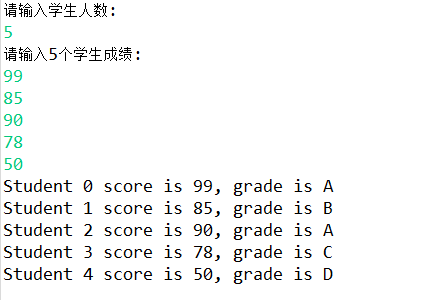
Scanner scanner = new Scanner(System.in);
2、循环读取输入:使用while (true)
循环不断提示用户输入,直到输入正确的非负正整数为止。
while (true) { try { System.out.print("请输入一个非负正整数:"); num = scanner.nextInt(); if (num > 0) { break; } else { System.out.println("输入的数不是非负正整数,请重新输入。"); } } catch (InputMismatchException e) { System.out.println("输入异常:" + e.toString()); scanner.next(); // 读取并丢弃错误的输入 } }
3、错误处理:如果用户输入的不是整数,会抛出InputMismatchException
异常,此时通过catch
块捕获异常并提示用户重新输入,调用scanner.next()
方法读取并丢弃错误的输入,避免死循环。
catch (InputMismatchException e) { System.out.println("输入异常:" + e.toString()); scanner.next(); // 读取并丢弃错误的输入 }
4、计算阶乘:当用户输入合法的非负正整数后,调用递归方法factorial
计算该数的阶乘,并输出结果。
System.out.println("您输入的非负正整数是:" + num); System.out.println("这个数的阶乘是:" + factorial(num));
5、关闭Scanner对象:关闭Scanner
对象以释放资源。
scanner.close();
常见问题解答(FAQs)
Q1:nextInt()
方法报错的原因是什么?
A1:nextInt()
方法报错通常是因为用户输入的数据类型不正确,如果用户输入了一个字符串而不是整数,nextInt()
方法会抛出InputMismatchException
异常,如果在调用nextInt()
之前已经调用了nextLine()
方法读取了整行数据,那么nextInt()
可能会无法正确读取整数,因为它期望读取的是下一个完整的整数输入。
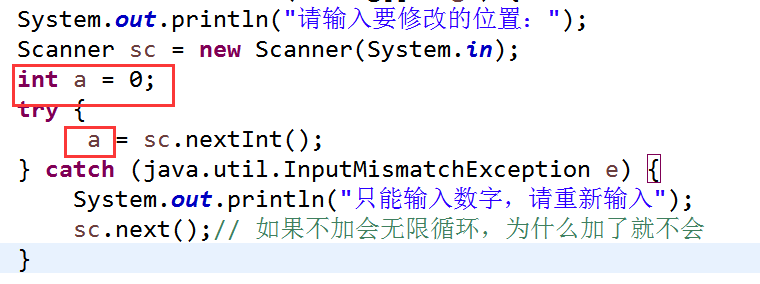
Q2: 如何避免nextInt()
方法报错?
A2: 为了避免nextInt()
方法报错,可以采取以下措施:
1、确保用户输入的数据类型正确,可以在调用nextInt()
之前使用hasNextInt()
方法检查输入是否为整数。
if (scanner.hasNextInt()) { num = scanner.nextInt(); } else { System.out.println("请输入一个整数。"); scanner.next(); // 读取并丢弃错误的输入 }
2、如果需要混合读取整数和字符串,可以先使用next()
方法读取整个输入行,然后使用字符串处理方法解析出需要的整数部分,这样可以避免由于换行符导致的读取问题。
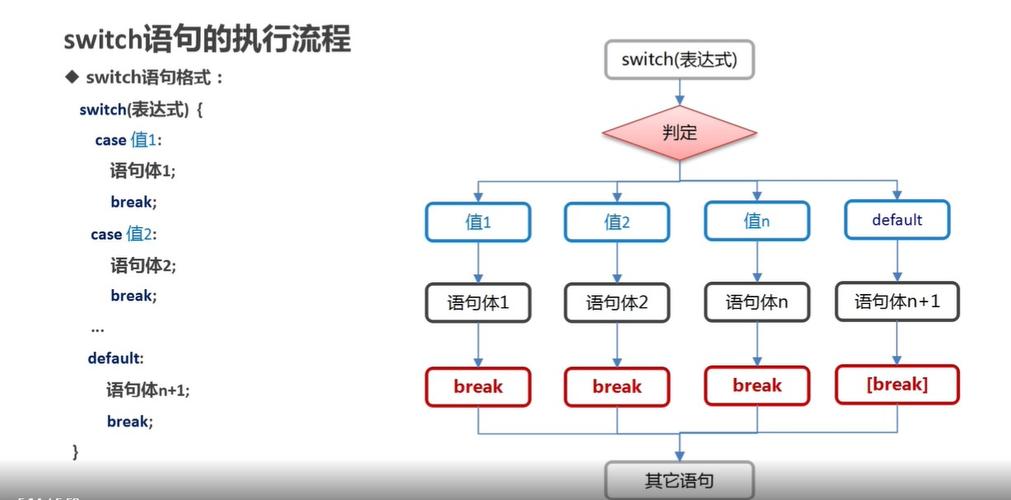