关于stringisempty
报错的全面解答
在Java编程中,处理字符串是否为空是一个常见的需求,当使用String.isEmpty()
方法时,如果字符串为null
,会抛出NullPointerException
错误,本文将详细解释这一问题的原因、解决方法以及相关的工具和最佳实践。
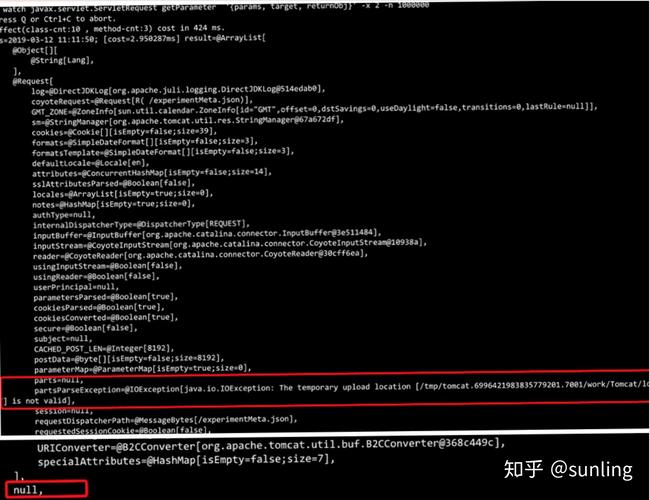
问题背景
在使用Java进行字符串操作时,经常需要判断一个字符串是否为空,通常的做法是使用String.isEmpty()
方法,但这个方法有一个潜在的问题:如果字符串对象本身是null
,则调用该方法会抛出NullPointerException
。
String str = null; if (str.isEmpty()) { // Do something }
上述代码会在运行时抛出以下异常:
java.lang.NullPointerException: Attempt to invoke virtual method 'boolean java.lang.String.isEmpty()' on a null object reference
这是因为isEmpty()
方法依赖于字符串对象的存在,而当字符串对象为null
时,它无法调用任何方法。
解决方案
为了安全地判断字符串是否为空,可以采用以下几种方法:
1、手动检查null
值
最简单的方法是先检查字符串是否为null
,然后再调用isEmpty()
方法。
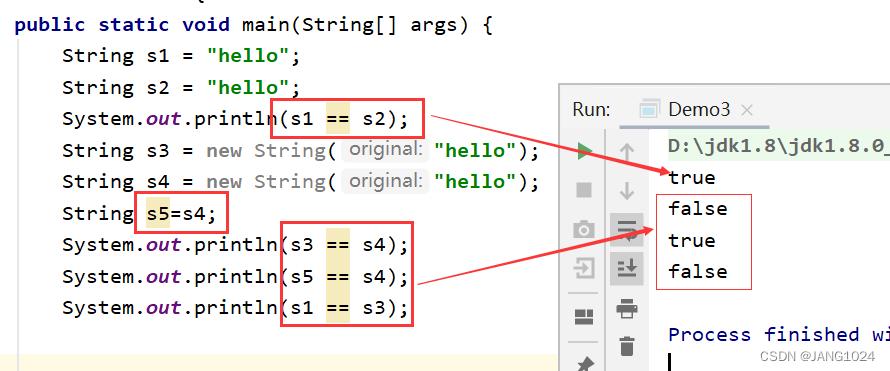
String str = null; if (str != null && !str.isEmpty()) { // The string is not null and not empty } else { // The string is null or empty }
2、使用Apache Commons Lang库中的StringUtils
类
Apache Commons Lang 提供了一个非常实用的StringUtils
类,其中包含了多个用于字符串操作的方法,包括isEmpty()
和isBlank()
,这些方法对null
值进行了处理,不会抛出NullPointerException
。
import org.apache.commons.lang3.StringUtils; String str = null; if (StringUtils.isEmpty(str)) { // The string is null or empty } else { // The string is not null and not empty }
3、使用Spring框架中的StringUtils
类
如果你使用的是Spring框架,也可以利用Spring提供的StringUtils
类来实现相同的功能。
import org.springframework.util.StringUtils; String str = null; if (!StringUtils.hasText(str)) { // The string is null, empty, or only whitespace } else { // The string has text }
常见误区与注意事项
区分null
、空字符串和空白字符串
null
:表示没有引用任何对象。
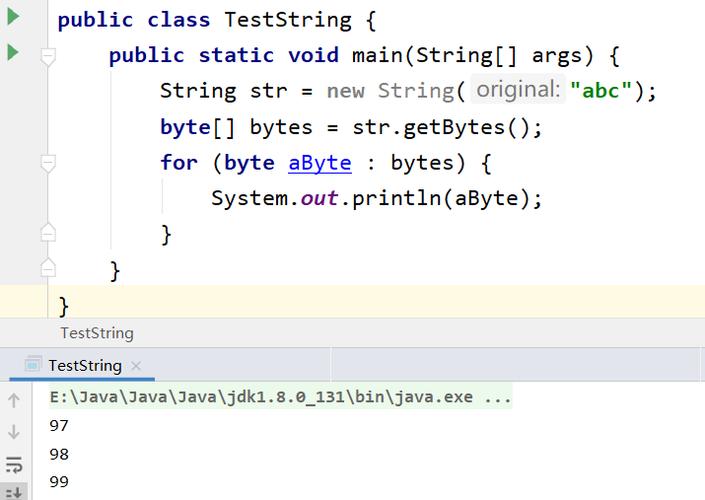
空字符串(""):表示长度为0的字符串。
空白字符串:包含空格、制表符等不可见字符的字符串。
在实际应用中,根据具体需求选择合适的判断方法,有时需要忽略空白字符,这时可以使用isBlank()
方法。
选择合适的工具类
不同的工具类提供了不同的方法来处理字符串,选择适合自己项目的工具类非常重要,如果你已经在项目中使用了Spring框架,那么使用Spring的StringUtils
会更加方便;如果没有引入第三方库,手动检查也是可行的方案。
最佳实践建议
1、优先使用成熟的库
尽量使用经过广泛测试和维护的第三方库,如Apache Commons Lang或Spring框架中的辅助类,以减少自己编写代码的工作量并提高代码的可靠性。
2、保持一致性
在整个项目中保持一致的字符串处理方法,避免混用不同的方法导致代码难以维护,可以选择在所有地方都使用StringUtils.isEmpty()
来判断字符串是否为空。
3、注意性能
虽然使用第三方库可以提高开发效率,但也要注意其对性能的影响,对于高性能要求的场景,应进行性能测试并根据结果做出调整。
4、编写单元测试
为字符串处理方法编写单元测试,确保在不同情况下都能正确工作,这有助于在修改代码后快速发现潜在问题。
相关FAQs
Q1: 为什么String.isEmpty()
在字符串为null
时报空指针异常?
A1:String.isEmpty()
方法依赖于字符串对象的存在,当字符串对象为null
时,尝试调用该方法会导致程序试图访问一个不存在的对象,从而抛出NullPointerException
,这是因为isEmpty()
方法内部实际上是通过调用value.length == 0
来判断字符串是否为空,而当字符串对象为null
时,无法访问其value
属性。
Q2: 如何在Java中安全地判断字符串是否为空?
A2: 可以通过以下几种方式安全地判断字符串是否为空:
手动检查null
值:先检查字符串是否为null
,然后再调用isEmpty()
方法。
String str = null; if (str != null && !str.isEmpty()) { // The string is not null and not empty } else { // The string is null or empty }
使用Apache Commons Lang库中的StringUtils
类:该类的isEmpty()
和isBlank()
方法对null
值进行了处理,不会抛出NullPointerException
。
import org.apache.commons.lang3.StringUtils; String str = null; if (StringUtils.isEmpty(str)) { // The string is null or empty } else { // The string is not null and not empty }
使用Spring框架中的StringUtils
类:该类的hasText()
方法可以判断字符串是否有文本内容(即非null
、非空且不含仅空白字符)。
import org.springframework.util.StringUtils; String str = null; if (!StringUtils.hasText(str)) { // The string is null, empty, or only whitespace } else { // The string has text }
选择适合自己项目的方法,确保代码的安全性和可读性。