hasownprototype报错分析与解决指南
在JavaScript编程中,hasOwnProperty
方法是一个常用的工具,用于检查对象是否直接拥有某个属性(即该属性不是从原型链继承来的),当使用这个方法时,如果遇到报错,可能会让人感到困惑,本文将详细分析hasOwnProperty
报错的原因,并提供相应的解决方案。
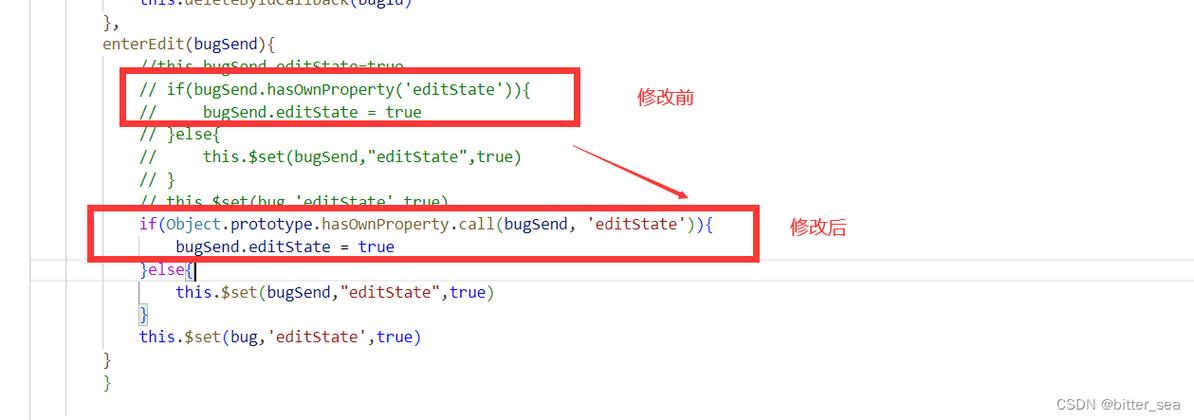
一、hasOwnProperty
方法简介
hasOwnProperty
是JavaScript对象的一个方法,它返回一个布尔值,指示对象自身(不包括其原型链)是否有指定的属性,语法如下:
object.hasOwnProperty(propertyName)
object
:要检查的对象。
propertyName
:要检查的属性名称,以字符串形式给出。
二、常见报错及原因分析
1. TypeError: object is not a function
这个错误通常出现在尝试调用非函数对象作为函数时。

var obj = {}; obj.hasOwnProperty(); // TypeError: object is not a function
原因:hasOwnProperty
是一个方法,需要在其所属的对象上调用,并且需要传入一个参数(属性名)。
解决方案:确保在正确的上下文中调用该方法,并传入必要的参数。
var obj = { name: 'Alice' }; console.log(obj.hasOwnProperty('name')); // true
2. TypeError: undefined is not an object
这个错误发生在尝试对undefined
或null
调用方法时。
var obj; console.log(obj.hasOwnProperty('name')); // TypeError: undefined is not an object
原因:undefined
和null
都不是对象,因此不能调用对象的方法。
解决方案:在使用hasOwnProperty
之前,先检查对象是否存在且不为null
。

var obj = null; if (obj !== null && obj !== undefined) { console.log(obj.hasOwnProperty('name')); } else { console.log('Object is null or undefined'); }
三、正确使用hasOwnProperty
的示例
为了进一步说明如何正确使用hasOwnProperty
,以下是一些示例代码:
// 示例1:基本用法 var person = { name: 'Alice', age: 30 }; console.log(person.hasOwnProperty('name')); // true console.log(person.hasOwnProperty('gender')); // false // 示例2:检查原型链上的属性 var animal = { species: 'Cat' }; var cat = Object.create(animal); cat.name = 'Whiskers'; console.log(cat.hasOwnProperty('name')); // true console.log(cat.hasOwnProperty('species')); // false, 因为是从原型继承来的 // 示例3:在数组中使用 var arr = [1, 2, 3]; arr.push = function() { console.log('Array push method overridden'); }; console.log(arr.hasOwnProperty('push')); // true, 因为push被重新定义了
四、相关问答FAQs
Q1:hasOwnProperty
与in
操作符有什么区别?
A1:hasOwnProperty
只检查对象自身是否包含某个属性,而忽略原型链中的属性,而in
操作符会检查对象及其原型链中是否包含某个属性,对于继承自原型的属性,hasOwnProperty
会返回false
,而in
会返回true
。
var animal = { species: 'Dog' }; var dog = Object.create(animal); dog.name = 'Buddy'; console.log(dog.hasOwnProperty('species')); // false console.log('species' in dog); // true
Q2: 如何安全地遍历对象的所有可枚举属性(包括原型链上的)?
A2: 可以使用for...in
循环来遍历对象的所有可枚举属性,包括原型链上的属性,但为了避免遍历到原型链上的属性,可以在循环内部使用hasOwnProperty
进行过滤。
var animal = { species: 'Elephant' }; var elephant = Object.create(animal); elephant.name = 'Dumbo'; elephant.age = 10; for (var key in elephant) { if (elephant.hasOwnProperty(key)) { console.log(key + ': ' + elephant[key]); // 只输出自有属性 } }
通过上述方法和注意事项,可以有效地避免在使用hasOwnProperty
时出现报错,并正确地利用它来检查对象的属性。