函数声明在编程中是至关重要的,它告诉编译器函数的存在和其参数类型,以便正确处理函数调用,函数声明错误会导致编译失败或运行时错误,下面将详细分析函数声明报错的原因及解决方法,并提供两个常见问题的解答:
函数声明报错的原因及解决方法
1、括号缺失:在C语言中,形参列表必须放在圆括号内。
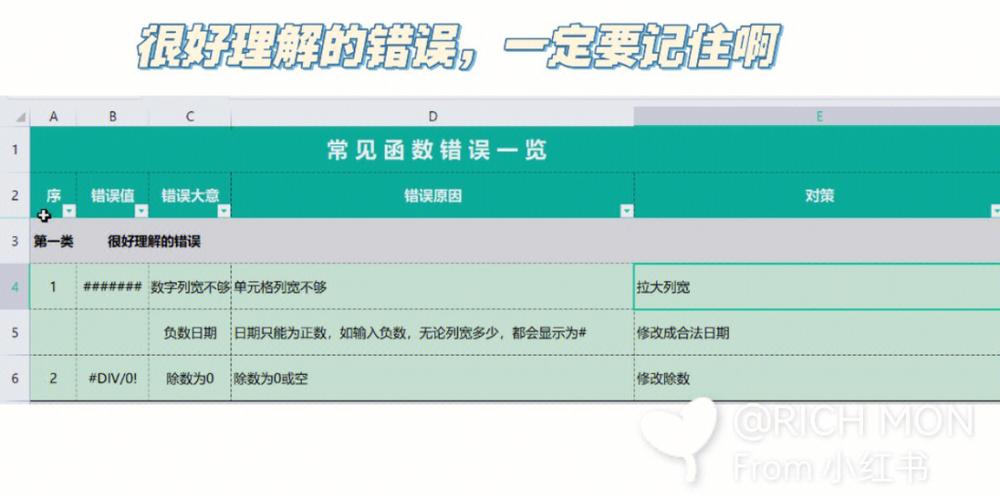
```c
int myFunction(int param1, int param2); // 正确
int myFunction int param1, int param2; // 错误,缺少括号
```
2、类型定义缺失:每个形参都需要指定数据类型。
```c
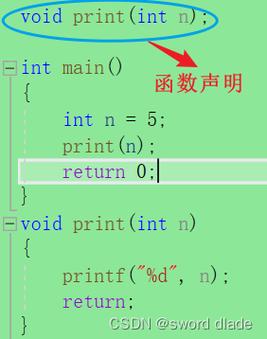
int myFunction(int, int); // 正确
int myFunction(int param1, param2); // 错误,第二个参数没有类型
```
3、逗号分隔符缺失:如果有多个形参,它们之间需要用逗号分隔。
```c
int myFunction(int param1 int param2); // 错误,缺少逗号
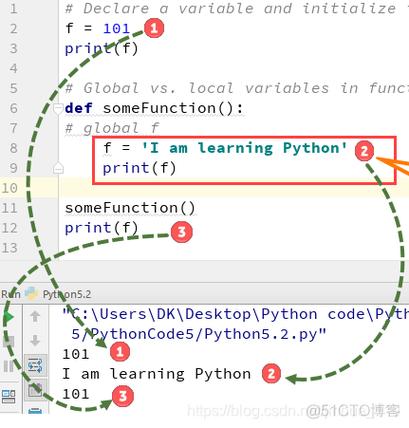
int myFunction(int param1, int param2); // 正确
```
4、变量名未定义:形参需要有名称,除非是void类型的函数。
```c
int myFunction(void); // 正确
int myFunction(); // 错误,没有明确指出参数列表为空
```
5、重复定义:如果同一个函数在多个文件中被定义,会导致重复定义错误。
```c
// a.h
#ifndef __a_h__
#define __a_h__
void funcA(void); // 声明
void funcA(void) {} // 定义
#endif
// b.h
#ifndef __b_h__
#define __b_h__
void funcB(void);
#endif
// b.cpp
#include "b.h"
#include "a.h"
void funcB(void) {
funcA();
}
// c.h
#ifndef __c_h__
#define __c_h__
void funcC(void);
#endif
// c.cpp
#include "c.h"
#include "a.h"
void funcC(void) {
funcA();
}
// main.cpp
#include "b.h"
#include "c.h"
int main(int argc, char* argv[]) {
funcB();
funcC();
return 0;
}
```
6、头文件包含顺序:头文件的包含顺序可能导致重复定义错误。
```c
// a.h
#ifndef __a_h__
#define __a_h__
void funcA(void); // 声明
void funcA(void) {} // 定义
#endif
// main.cpp
#include "a.h"
#include "main.h"
```
7、作用域问题:确保函数声明在其使用的作用域内可见。
```c
// main.cpp
#include "myheader.h"
void someFunction() {
myFunction(1, 2); // 确保myFunction在此作用域内声明过
}
```
8、包含正确的库文件:对于第三方库中的函数,确保包含了正确的头文件。
```c
#include <some_library.h> // 确保包含了正确的头文件
void useLibraryFunction() {
libraryFunction(); // 使用库函数
}
```
FAQs(常见问题解答)
1、为什么在头文件里声明函数会这样报错?
回答:在头文件里声明函数时,常见的错误包括括号缺失、类型定义缺失、逗号分隔符缺失以及变量名未定义等,这些错误会导致编译器无法识别函数声明,从而报错,确保函数声明遵循正确的语法格式,并在需要的地方包含正确的头文件。
2、如何解决“implicit declaration of function”错误?
回答:当编译器报“implicit declaration of function”错误时,通常是因为函数在使用前没有被声明,解决方法包括:检查拼写和大小写是否正确;在使用函数之前显式地声明它;确保函数在当前作用域内可见;包含正确的库文件;清理编译选项,确保没有禁用隐含声明;升级到最新版编译器,通过这些步骤,可以解决大多数“implicit declaration of function”错误。
通过上述内容,可以全面理解函数声明报错的原因及解决方法,并掌握解决常见问题的技巧。