在使用Apache POI库进行Excel文件操作时,经常会遇到一些报错问题,这些问题通常与API的变更、版本兼容性以及代码实现细节有关,下面将详细分析HSSFCellStyle报错问题,并提供解决方案和相关示例代码。
HSSFCellStyle报错问题及解决方案
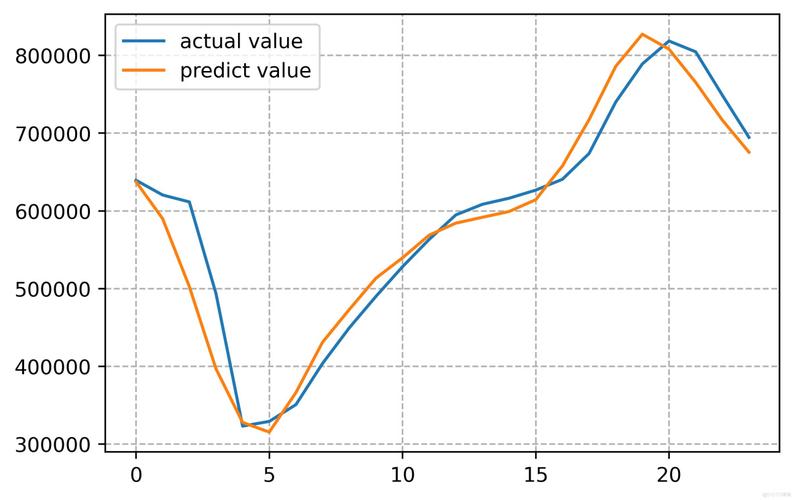
1、HSSFCellStyle.ALIGN_CENTER报错:这是因为在新版本的POI中,HSSFCellStyle.ALIGN_CENTER
已经被弃用,需要使用HorizontalAlignment.CENTER
来代替。
HSSFCellStyle cellStyle = workbook.createCellStyle(); cellStyle.setAlignment(HorizontalAlignment.CENTER); // 水平居中
2、HSSFColor.GREY_25_PERCENT.index报错:同样地,HSSFColor.GREY_25_PERCENT.index
也需要替换为IndexedColors.GREY_25_PERCENT.getIndex()
。
cellStyle.setFillBackgroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
3、HSSFCellStyle.SOLID_FOREGROUND报错:HSSFCellStyle.SOLID_FOREGROUND
需要替换为FillPatternType.SOLID_FOREGROUND
。
cellStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND);
4、HSSFFont.BOLDWEIGHT_BOLD报错:HSSFFont.BOLDWEIGHT_BOLD
应改为调用setBold(true)
方法。
HSSFFont font = workbook.createFont(); font.setBold(true); cellStyle.setFont(font);
5、XSSFCell.CELL_TYPE_STRING报错:XSSFCell.CELL_TYPE_string
需要替换为CellType.STRING
。
Cell cell = row.createCell(column, CellType.STRING);
6、HSSFCellStyle超出最大数限制:每个Workbook创建的CellStyle有最大数限制,xls的最大数是4000,.xlsx的最大数是64000,如果超过这个限制,会抛出IllegalStateException
异常,解决方案包括实现单元格样式的复用或将createCellStyle放在循环外面。
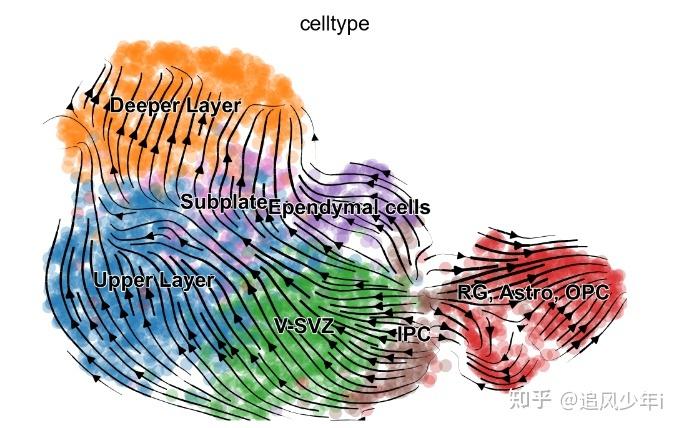
方案1:缓存样式实现复用
Workbook workBook = new HSSFWorkbook(); Sheet sheet = workBook.createSheet("strSheetName"); Map<String, CellStyle> cellStyleMap = new HashMap<>(); for (int rowIndex = 0; rowIndex < maxRow; rowIndex++) { Row row = sheet.createRow(rowIndex); for (int colIndex = 0; colIndex < maxCol; colIndex++) { Cell cell = row.createCell((short) colIndex); String styKey = getCellStyleKey(rowIndex, colIndex); CellStyle cellStyle = cellStyleMap.computeIfAbsent(styKey, k > workBook.createCellStyle()); cell.setCellStyle(cellStyle); } }
方案2:修改限制参数(不推荐)
示例代码
以下是一个完整的示例代码,展示了如何使用Apache POI库创建一个新的Excel文件并设置单元格样式:
import org.apache.poi.ss.usermodel.*; import org.apache.poi.xssf.usermodel.XSSFWorkbook; import java.io.FileOutputStream; import java.io.IOException; public class ExcelExample { public static void main(String[] args) throws IOException { Workbook workbook = new XSSFWorkbook(); Sheet sheet = workbook.createSheet("Sheet1"); // 创建单元格样式 CellStyle cellStyle = workbook.createCellStyle(); cellStyle.setAlignment(HorizontalAlignment.CENTER); // 水平居中 cellStyle.setVerticalAlignment(VerticalAlignment.CENTER); // 垂直居中 cellStyle.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex()); // 设置背景颜色 cellStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND); // 填充模式 Font font = workbook.createFont(); font.setBold(true); cellStyle.setFont(font); // 创建一行 Row row = sheet.createRow(0); // 创建一个单元格并应用样式 Cell cell = row.createCell(0, CellType.STRING); cell.setCellValue("Hello, World!"); cell.setCellStyle(cellStyle); // 写入Excel文件 try (FileOutputStream fileOut = new FileOutputStream("example.xlsx")) { workbook.write(fileOut); } workbook.close(); } }
在使用Apache POI进行Excel文件操作时,遇到hssfCellStyle
报错通常是由于API的变更或版本兼容性问题,通过更新代码以适应新的API,可以解决大部分问题,注意每个Workbook创建的CellStyle有最大数限制,可以通过实现单元格样式的复用来避免超出限制,希望以上内容能够帮助开发者更好地理解和解决在使用Apache POI时遇到的问题。
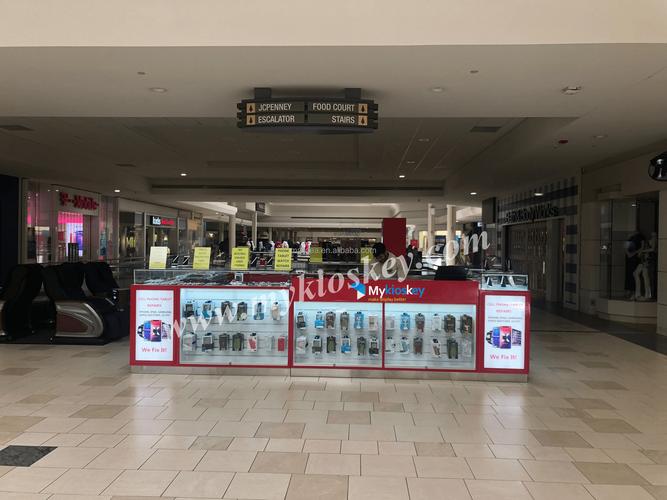