mounted报错
在使用Vue.js框架进行前端开发时,mounted
是组件生命周期钩子之一,它在组件被挂载到DOM之后立即调用,开发者在实现这个钩子时可能会遇到各种错误,本文将详细探讨常见的mounted
报错原因及其解决方案。

1.mounted
报错的常见原因与解决方案
1.1 未定义mounted
钩子
错误信息:
[Vue warn]: Property or method "mounted" is not defined on the instance but referenced during render. Make sure to declare reactive data properties in the component's data option.
原因:
在Vue实例或组件中没有正确声明mounted
钩子。
解决方案:
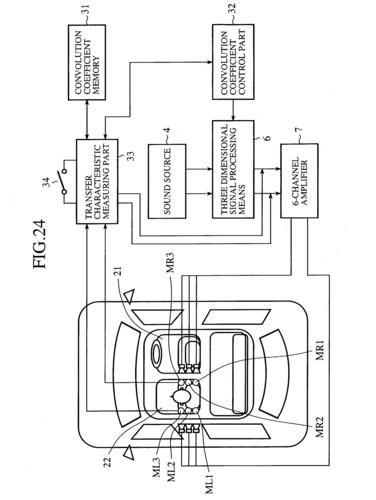
确保在Vue组件中正确声明mounted
钩子。
export default { name: 'MyComponent', mounted() { // Your code here } }
1.2 异步操作未处理
错误信息:
Uncaught (in promise) TypeError: Cannot read property 'then' of undefined
原因:
在mounted
钩子中使用了异步操作(如Ajax请求),但未正确处理Promise。
解决方案:
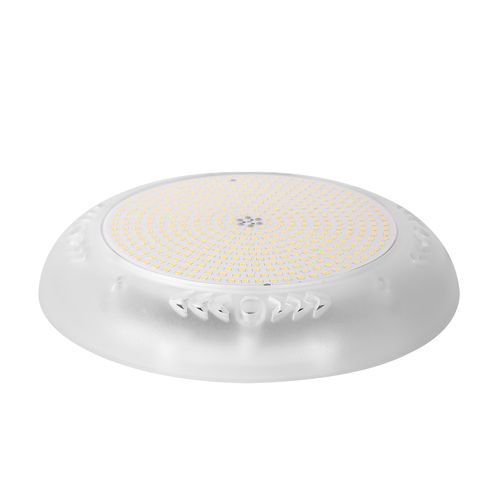
使用async/await
语法或.then().catch()
方法来处理异步操作。
export default { name: 'MyComponent', mounted() { this.fetchData(); }, methods: { async fetchData() { try { const response = await axios.get('https://api.example.com/data'); this.data = response.data; } catch (error) { console.error('Error fetching data:', error); } } } }
1.3 依赖项未初始化
错误信息:
TypeError: Cannot read property 'someProperty' of undefined
原因:
mounted
钩子依赖于某些尚未初始化的数据或属性。
解决方案:
确保在mounted
钩子执行前,所有依赖项都已正确初始化,可以在data
或computed
中预先设置默认值。
export default { name: 'MyComponent', data() { return { someProperty: null, }; }, mounted() { if (this.someProperty) { // Do something with this.someProperty } else { console.error('someProperty is not initialized'); } } }
1.4 访问DOM元素失败
错误信息:
TypeError: Cannot read property 'value' of undefined
原因:
尝试在mounted
钩子中访问尚未渲染的DOM元素。
解决方案:
确保在DOM元素完全渲染后再访问它们,可以使用$nextTick
来确保在下一次DOM更新循环后执行代码。
export default { name: 'MyComponent', mounted() { this.$nextTick(() => { const inputElement = this.$refs.myInput; if (inputElement) { console.log(inputElement.value); } else { console.error('Input element not found'); } }); } }
1.5 第三方库错误
错误信息:
Error: [thirdpartylibrary] Something went wrong
原因:
使用了有错误的第三方库或插件。
解决方案:
检查第三方库的文档和常见问题解答,确保正确安装和使用,如果问题依然存在,可以尝试更新到最新版本或寻求社区帮助。
npm update thirdpartylibrary
相关问答FAQs
Q1:mounted
钩子中的代码为什么有时不会执行?
A1:mounted
钩子中的代码不会执行的原因可能有多种,包括但不限于以下几点:
组件未正确挂载到DOM。
组件被其他条件渲染指令(如vif
)控制,且条件不满足。
组件被异步加载,且在mounted
钩子执行时还未完成加载。
要排查这个问题,可以检查组件是否正确挂载,以及相关的渲染条件是否满足。
Q2: 如何在mounted
钩子中安全地访问子组件的引用?
A2: 在mounted
钩子中访问子组件的引用时,需要确保子组件已经渲染并可用,可以使用$refs
来获取子组件的引用,并在$nextTick
回调函数中访问它们。
export default { name: 'ParentComponent', mounted() { this.$nextTick(() => { const childComponent = this.$refs.childComponent; if (childComponent) { // Safely access childComponent's methods or properties childComponent.someMethod(); } else { console.error('Child component not found'); } }); } }
这样可以确保在子组件完全渲染后再进行访问,避免出现未定义的错误。