在java编程中,isEmpty()
方法通常用于检查集合是否为空,当调用该方法的对象为null
时,会抛出NullPointerException
异常,这是因为isEmpty()
方法需要一个非空的集合对象才能正确执行。
常见场景

1、字符串操作:在使用String
类的isEmpty()
方法时,如果字符串对象为null
,则会触发NullPointerException
。
2、集合操作:类似地,对于List
、Set
等集合类,如果集合对象为null
,调用isEmpty()
方法也会导致相同的异常。
报错原因分析
场景 | 错误描述 | 原因 |
String | queryById.getUserSponsortwo().isEmpty() | 当queryById.getUserSponsortwo() 返回null 时,调用isEmpty() 会抛出NullPointerException |
List | mapList.isEmpty() | 如果mapList 未初始化或为null ,则调用isEmpty() 会抛出NullPointerException |
解决方案
1、使用工具类:可以使用apache Commons Lang库中的StringUtils
类来避免空指针异常,使用StringUtils.isBlank()
或StringUtils.isEmpty()
方法来判断字符串是否为空。
2、先判空再调用:在调用isEmpty()
之前,先检查对象是否为null
,如果是null
,则直接处理或返回特定值。

3、使用第三方库:如Spring框架中的CollectionUtils.isEmpty()
方法,它在内部进行了null
检查,可以避免空指针异常。
示例代码
字符串判断
- import org.apache.commons.lang3.StringUtils;
- public class StringExample {
- public static void main(String[] args) {
- String str = null;
- if (StringUtils.isBlank(str)) {
- System.out.println("The string is null or empty");
- } else {
- System.out.println("The string is not null and not empty");
- }
- }
- }
集合判断
- import java.util.ArrayList;
- import java.util.List;
- import org.springframework.util.CollectionUtils;
- public class CollectionExample {
- public static void main(String[] args) {
- List<String> list = null;
- if (CollectionUtils.isEmpty(list)) {
- System.out.println("The list is null or empty");
- } else {
- System.out.println("The list is not null and not empty");
- }
- }
- }
在使用isEmpty()
方法前,务必确保对象不为null
,以避免空指针异常,可以通过使用工具类或进行前置检查来实现这一点,这样不仅可以提高代码的健壮性,还能有效避免运行时错误。
FAQs
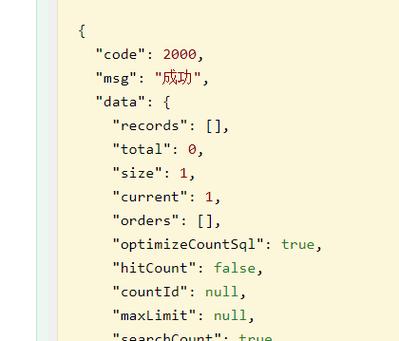
1、为什么isEmpty()
方法会在对象为null
时抛出异常?
isEmpty()
方法要求对象必须已经实例化,即不能为null
,当对象为null
时,JVM不知道该如何处理这个引用,因此会抛出NullPointerException
。
2、如何安全地检查字符串是否为空?
可以使用Apache Commons Lang库中的StringUtils.isBlank()
或StringUtils.isEmpty()
方法来安全地检查字符串是否为空,这些方法在内部进行了null
检查。