在处理文件或数据时,如果遇到与编码相关的问题,尤其是涉及到读取 UTF8 编码的文件时,可能会遇到报错,本文将详细解析readutf
函数的常见错误及其解决方案,并提供一些常见问题解答(FAQs)。
常见报错及解决方案
1. 错误类型:UnicodeDecodeError
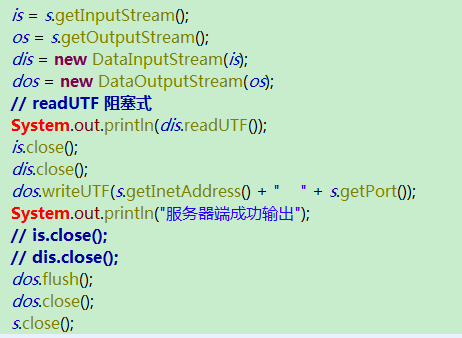
描述:这个错误通常发生在尝试以错误的编码方式读取文件时,文件实际是 UTF8 编码的,但读取时使用了 ASCII 或其他不兼容的编码方式。
示例:
- with open('example.txt', 'r') as file:
- content = file.read()
解决方案:
确保使用正确的编码方式打开文件,对于 UTF8 编码的文件,可以使用encoding='utf8'
参数。
- with open('example.txt', 'r', encoding='utf8') as file:
- content = file.read()
2. 错误类型:FileNotFoundError
描述:当指定的文件路径不存在时,会引发此错误。
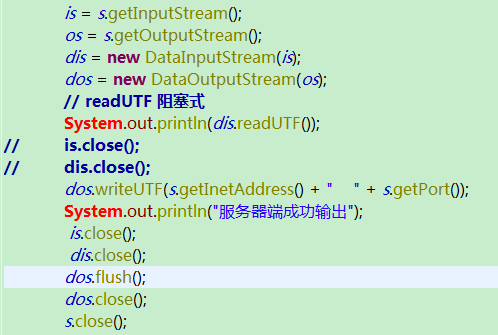
示例:
- with open('non_existent_file.txt', 'r', encoding='utf8') as file:
- content = file.read()
解决方案:
确保指定的文件路径正确且文件存在。
- import os
- if os.path.exists('example.txt'):
- with open('example.txt', 'r', encoding='utf8') as file:
- content = file.read()
- else:
- print("The file does not exist.")
3. 错误类型:IsADirectoryError
描述:当尝试打开一个目录而不是文件时,会引发此错误。
示例:
- with open('/path/to/directory', 'r', encoding='utf8') as file:
- content = file.read()
解决方案:
确保指定的路径是一个文件,而不是目录。
- import os
- if os.path.isfile('/path/to/file'):
- with open('/path/to/file', 'r', encoding='utf8') as file:
- content = file.read()
- elif os.path.isdir('/path/to/directory'):
- print("The specified path is a directory, not a file.")
- else:
- print("The specified path does not exist.")
错误类型 | 描述 | 解决方案 |
UnicodeDecodeError | 文件实际是 UTF8 编码,但读取时使用了不兼容的编码方式 | 确保使用正确的编码方式打开文件,如encoding='utf8' |
FileNotFoundError | 指定的文件路径不存在 | 确保指定的文件路径正确且文件存在 |
IsADirectoryError | 尝试打开一个目录而不是文件 | 确保指定的路径是一个文件,而不是目录 |
常见问题解答(FAQs)
Q1:如何确定文件的编码方式?
A1:可以使用第三方库如chardet
来检测文件的编码方式,安装chardet
后,可以使用以下代码检测文件编码:
- import chardet
- with open('example.txt', 'rb') as file:
- rawdata = file.read()
- result = chardet.detect(rawdata)
- encoding = result['encoding']
- print(f"Detected encoding: {encoding}")
Q2:如何处理包含非 UTF8 字符的文件?
A2:在读取文件时,可以指定忽略错误或使用替代字符来处理非 UTF8 字符。
- with open('example.txt', 'r', encoding='utf8', errors='ignore') as file:
- content = file.read()
- 或者使用替代字符
- with open('example.txt', 'r', encoding='utf8', errors='replace') as file:
- content = file.read()
通过以上方法,可以有效解决readutf
函数在读取文件时遇到的常见报错问题,希望这些内容对你有所帮助。