在编程中,each
通常是一个用于迭代集合(如列表、数组、字典等)的方法,不同的编程语言和框架可能有不同的实现方式,下面将详细介绍几种常见语言中的each
方法及其报错处理,并给出相应的示例代码和解决方案。
Ruby 中的 each
1.1 基本用法
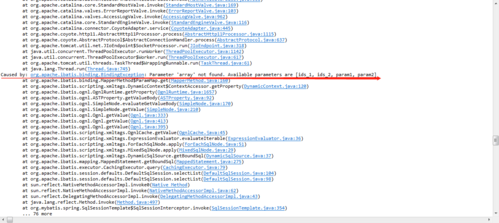
arr = [1, 2, 3] arr.each do |item| puts item end
1.2 常见错误及解决方案
1.2.1 错误:未定义变量
问题描述:NameError: undefined local variable or method 'x' for main:Object
原因: 变量x
未被定义或初始化。
解决方案: 确保在使用each
之前已经正确定义并初始化了变量。
x = [1, 2, 3] x.each do |item| puts item end
1.2.2 错误:非数组或非枚举对象调用each

问题描述:TypeError: no block given (yield)
原因: 对一个非数组或非枚举对象调用each
方法。
解决方案: 确保调用each
的对象是可迭代的。
arr = [1, 2, 3] arr.each do |item| puts item end
JavaScript 中的 each
2.1 基本用法
let arr = [1, 2, 3]; arr.forEach(function(item) { console.log(item); });
2.2 常见错误及解决方案
2.2.1 错误:未定义变量
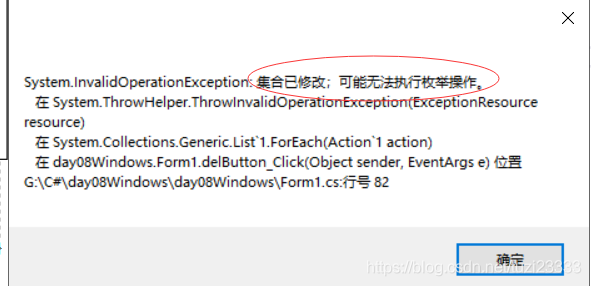
问题描述:ReferenceError: x is not defined
原因: 变量x
未被定义或初始化。
解决方案: 确保在使用forEach
之前已经正确定义并初始化了变量。
let x = [1, 2, 3]; x.forEach(function(item) { console.log(item); });
2.2.2 错误:非数组对象调用forEach
问题描述:TypeError: x.forEach is not a function
原因: 对一个非数组对象调用forEach
方法。
解决方案: 确保调用forEach
的对象是数组。
let arr = [1, 2, 3]; arr.forEach(function(item) { console.log(item); });
三、Python 中的 each(使用 for 循环)
3.1 基本用法
arr = [1, 2, 3] for item in arr: print(item)
3.2 常见错误及解决方案
3.2.1 错误:未定义变量
问题描述:NameError: name 'x' is not defined
原因: 变量x
未被定义或初始化。
解决方案: 确保在使用for
循环之前已经正确定义并初始化了变量。
x = [1, 2, 3] for item in x: print(item)
3.2.2 错误:非迭代对象使用for
循环
问题描述:TypeError: 'int' object is not iterable
原因: 对一个非迭代对象使用for
循环。
解决方案: 确保使用for
循环的对象是可迭代的。
arr = [1, 2, 3] for item in arr: print(item)
相关问答 FAQs
Q1: 如何在 Ruby 中处理each
方法的参数?
A1: 在 Ruby 中,each
方法会将当前元素传递给块中的局部变量。
arr = [1, 2, 3] arr.each do |item| puts "Current element: #{item}" end
如果希望同时获取索引,可以使用each_with_index
:
arr = [1, 2, 3] arr.each_with_index do |item, index| puts "Index: #{index}, Element: #{item}" end
Q2: 在 JavaScript 中如何终止forEach
循环?
A2: JavaScript 的forEach
方法没有直接提供终止循环的方法,但可以通过抛出异常来达到类似的效果,更推荐使用for
、while
或for...of
循环,这些循环支持break
:
let arr = [1, 2, 3]; for (let i = 0; i < arr.length; i++) { if (arr[i] === 2) { break; // 终止循环 } console.log(arr[i]); }