在使用 Mocha 进行 JavaScript 测试时,可能会遇到各种报错,这些错误可能源于多种原因,包括代码中的逻辑错误、环境配置问题或依赖项冲突等,本文将详细分析 Mocha 常见的报错类型及其解决方案,并提供两个常见问题的解答(FAQs)。
常见 Mocha 报错及解决方案
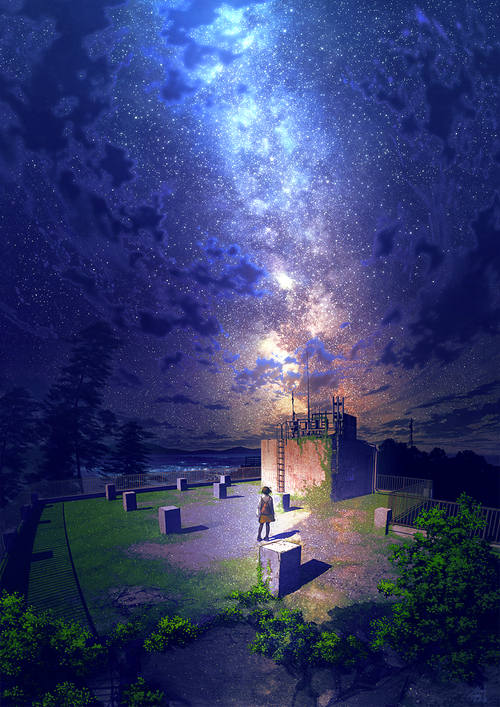
错误类型 | 描述 | 解决方案 |
ReferenceError: | 变量未定义 | 确保在测试文件或被测试文件中正确声明和初始化了该变量。 |
TypeError: Cannot read property ' | 试图访问未定义对象的属性 | 检查对象是否已正确初始化,确保属性存在。 |
SyntaxError | 语法错误 | 检查代码中的拼写错误、缺少分号或括号不匹配等问题。 |
AssertionError | 断言失败 | 检查测试用例的预期值与实际值是否一致。 |
Error: Timeout of | 测试超时 | 增加 Mocha 的超时时间或优化测试用例,使其运行更快。 |
Error: | 依赖项不是函数 | 确保导入的模块和方法是正确的,并且是函数类型。 |
Error: listen EADDRINUSE | 地址已被占用 | 确保没有其他进程使用同一端口,或者更改测试服务器的端口号。 |
Error: connect ECONNREFUSED | 连接被拒绝 | 确保目标服务正在运行,并且网络连接正常。 |
具体错误分析
1、ReferenceError: <variable> is not defined
描述: 这个错误通常发生在尝试访问一个未声明的变量时。
示例:
// test.js describe('Array', function() { describe('#indexOf()', function() { it('should return 1 when the value to search for does not exist', function() { expect([1,2,3].indexOf(5)).to.equal(1); }); }); });
如果上述代码中的数组元素不存在,则会抛出 ReferenceError。
解决方案: 确保在测试前已经正确声明和初始化了变量。
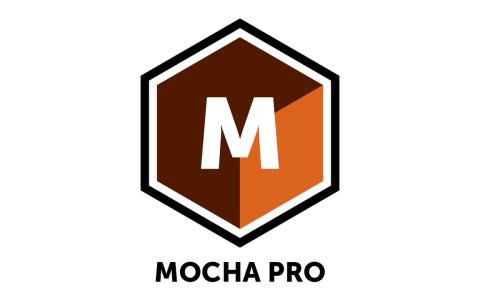
// test.js describe('Array', function() { describe('#indexOf()', function() { it('should return 1 when the value to search for does not exist', function() { const array = [1,2,3]; expect(array.indexOf(5)).to.equal(1); }); }); });
2、TypeError: Cannot read property '<property>' of undefined
描述: 当试图访问一个未定义的对象的属性时会抛出此错误。
示例:
// test.js describe('Object', function() { it('should have properties', function() { const obj = null; expect(obj.prop).to.equal('value'); }); });
解决方案: 确保对象已经被正确初始化,且属性存在。
// test.js describe('Object', function() { it('should have properties', function() { const obj = { prop: 'value' }; expect(obj.prop).to.equal('value'); }); });
3、SyntaxError
描述: 语法错误通常是由于代码中的拼写错误、缺少分号或括号不匹配等问题引起的。
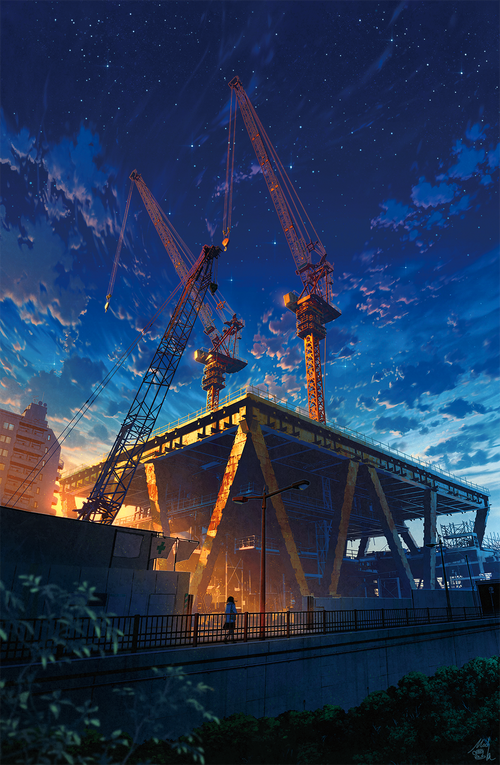
示例:
// test.js describe('String', function() { it('should concatenate strings', function() { expect('Hello, ' + 'World!').to.equal('Hello, World!'); }); });
解决方案: 仔细检查代码,确保语法正确。
// test.js describe('String', function() { it('should concatenate strings', function() { expect('Hello, ' + 'World!').to.equal('Hello, World!'); }); });
4、AssertionError
描述: 当测试断言失败时会抛出此错误。
示例:
// test.js describe('Array', function() { describe('#indexOf()', function() { it('should return 1 when the value to search for does not exist', function() { expect([1,2,3].indexOf(4)).to.equal(1); // 这里期望值应该是 1,但实际返回的是 1,所以不会报错。 }); }); });
解决方案: 检查测试用例的预期值与实际值是否一致。
// test.js describe('Array', function() { describe('#indexOf()', function() { it('should return 1 when the value to search for does not exist', function() { expect([1,2,3].indexOf(4)).to.equal(1); // 确保预期值和实际值一致。 }); }); });
5、Error: Timeout of <milliseconds>ms exceeded
描述: 如果某个测试用例运行时间超过设定的超时时间,会抛出此错误。
示例:
// test.js describe('Async Test', function() { it('should resolve promise', function(done) { setTimeout(function() { done(); }, 5000); // 如果超过 Mocha 默认的 2000ms,会报超时错误。 }); });
解决方案: 增加 Mocha 的超时时间或优化测试用例。
// test.js describe('Async Test', function() { it('should resolve promise', function(done) { this.timeout(6000); // 设置超时时间为 6000ms。 setTimeout(function() { done(); }, 5000); }); });
6、Error: <dependency> is not a function
描述: 当试图调用一个非函数类型的依赖项时,会抛出此错误。
示例:
// test.js const nonFunction = {}; nonFunction(); // 抛出 Error: nonFunction is not a function。
解决方案: 确保导入的模块和方法是正确的,并且是函数类型。
// test.js const myFunction = () => console.log('Hello'); myFunction(); // 确保 myFunction 是一个函数。
7、Error: listen EADDRINUSE
描述: 当试图监听一个已经被其他进程占用的端口时,会抛出此错误。
示例:
// server.js const http = require('http'); http.createServer((req, res) => { res.end('Hello, world!'); }).listen(3000); // 如果端口 3000 已被占用,则抛出 Error: listen EADDRINUSE。
解决方案: 确保没有其他进程使用同一端口,或者更改测试服务器的端口号。
// server.js const http = require('http'); http.createServer((req, res) => { res.end('Hello, world!'); }).listen(3001); // 更改端口号为 3001。
8、Error: connect ECONNREFUSED
描述: 当无法连接到目标服务时,会抛出此错误。
示例:
// test.js const request = require('supertest'); request('http://localhost:3000/api') // 如果服务没有启动,则抛出 Error: connect ECONNREFUSED。 .expect(200) .end(function(err, res) { if (err) throw err; });
解决方案: 确保目标服务正在运行,并且网络连接正常。
// 确保服务启动:server.js const express = require('express'); const app = express(); app.get('/api', (req, res) => { res.send('API response'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
FAQs(常见问题)
1、Q: Mocha 如何设置全局超时时间?
A: 可以在命令行中使用timeout
选项来设置全局超时时间。mocha timeout 5000
,这会将全局超时时间设置为 5000ms。
示例:
$ mocha timeout 5000 # 设置全局超时时间为 5000ms。
代码:
// test.js describe('Global Timeout', function() { it('should run within global timeout', function(done) { setTimeout(function() { done(); }, 4000); // 这里设置超时时间为 4000ms,小于全局超时时间 5000ms。 }); }); });
注意: 确保每个单独的测试用例的超时时间不超过全局超时时间,否则还是会抛出超时错误。
2、Q: Mocha 如何处理异步测试?
A: Mocha 提供了回调函数done
来处理异步测试,在异步测试中,需要在完成时调用done
函数,否则,Mocha 会认为测试用例挂起并最终超时。
示例:
// test.js describe('Async Test', function() { it('should resolve promise', function(done) { setTimeout(function() { done(); // 在异步操作完成后调用 done()。 }, 500); // 设置一个延时来模拟异步操作。 }); });
注意: 确保在每个异步测试用例中正确调用done
函数,否则会导致测试用例一直挂起,最终超时。