在JavaScript中,.length
属性通常用于获取数组或字符串的长度,如果你在非数组或非字符串对象上使用.length
属性,就可能会引发错误,下面将详细解释这个问题,并提供解决方案和示例。
问题描述
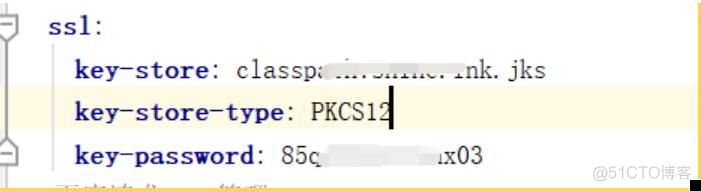
1、什么是.length
属性?
.length
是 JavaScript 中用于获取数组、字符串等集合类型的长度的属性。
let arr = [1, 2, 3]; console.log(arr.length); // 输出: 3 let str = "Hello"; console.log(str.length); // 输出: 5
2、什么情况下会报错?
当你尝试访问一个非数组或非字符串对象的.length
属性时,会引发错误。
let obj = {}; console.log(obj.length); // 报错: TypeError: Cannot read properties of undefined (reading 'length')
原因分析
1、非数组和非字符串对象没有内置的.length
属性:
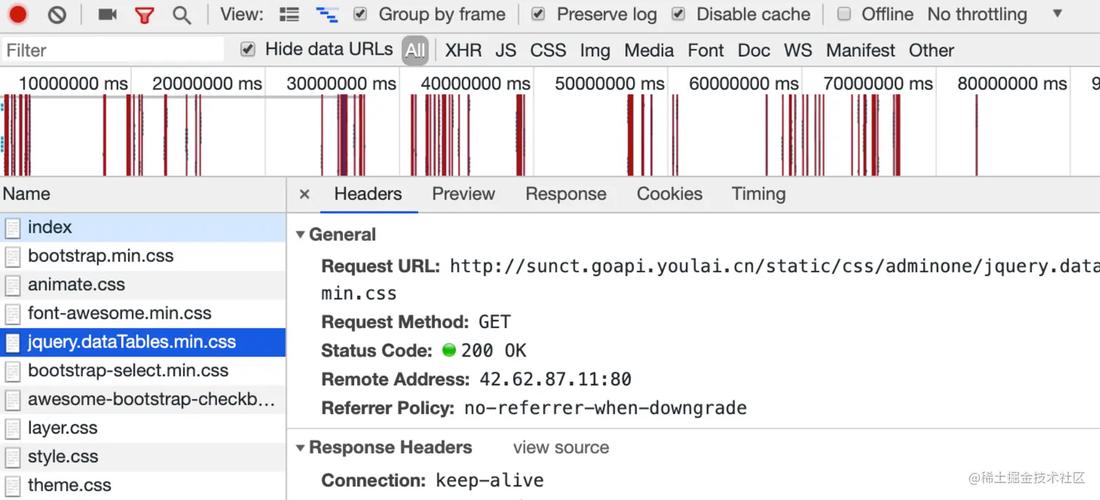
普通对象(plain objects)和其他数据类型(如数字、布尔值、函数等)都没有内置的.length
属性,当你试图访问这些类型的.length
属性时,会导致TypeError
。
2、如何判断对象类型?
在访问.length
属性之前,可以使用typeof
操作符或instanceof
操作符来判断对象类型:
if (Array.isArray(arr)) { console.log(arr.length); } else if (typeof str === 'string') { console.log(str.length); }
解决方案
1、使用Array.isArray()
检查是否是数组:
Array.isArray()
方法可以准确判断一个变量是否是数组:
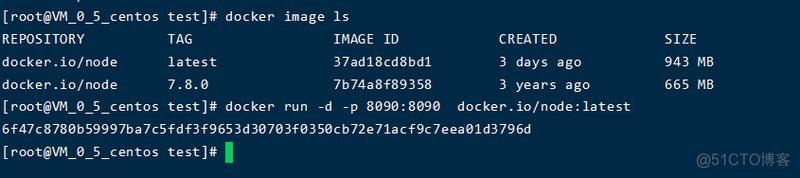
let data = [1, 2, 3]; if (Array.isArray(data)) { console.log(data.length); // 输出: 3 } else { console.log("Not an array"); }
2、使用typeof
操作符检查是否是字符串:
typeof
操作符可以用来判断一个变量是否是字符串:
let data = "Hello"; if (typeof data === 'string') { console.log(data.length); // 输出: 5 } else { console.log("Not a string"); }
3、综合判断多种情况:
结合上述两种方法,可以处理多种数据类型:
function getLength(data) { if (Array.isArray(data)) { return data.length; } else if (typeof data === 'string') { return data.length; } else { throw new Error("Unsupported data type for .length"); } } try { console.log(getLength([1, 2, 3])); // 输出: 3 console.log(getLength("Hello")); // 输出: 5 console.log(getLength({})); // 抛出错误: Unsupported data type for .length } catch (error) { console.error(error.message); }
示例表格
数据类型 | .length 是否可用 | 示例代码 | 结果 |
数组 | 是 | let arr = [1, 2, 3]; console.log(arr.length); | 输出: 3 |
字符串 | 是 | let str = "Hello"; console.log(str.length); | 输出: 5 |
对象 | 否 | let obj = {}; console.log(obj.length); | 报错: TypeError |
数字 | 否 | let num = 42; console.log(num.length); | 报错: TypeError |
布尔值 | 否 | let bool = true; console.log(bool.length); | 报错: TypeError |
函数 | 否 | function func() {}; console.log(func.length); | 输出: 函数参数个数 |
相关问答FAQs
Q1: 为什么在对象上使用.length
会报错?
A1: 对象(plain objects)没有内置的.length
属性,只有数组和字符串才有这个属性,在对象上使用.length
会导致TypeError
。
Q2: 如何安全地获取不同类型数据的.length
?
A2: 在访问.length
之前,应先判断数据类型,对于数组,可以使用Array.isArray()
;对于字符串,可以使用typeof
,确保数据类型正确后再访问.length
,以避免错误。