在多线程编程中,使用并发集合(如ConcurrentHashMap)时,经常会遇到putIfAbsent报错的问题,本文将详细解释putIfAbsent方法的工作原理、常见错误及解决方案,并提供相关代码示例和FAQs。
putIfAbsent方法
putIfAbsent方法是Java 5引入的一种原子性操作,用于在并发环境下安全地向Map中添加键值对,其基本逻辑是:如果指定的键不存在,则将键值对插入到Map中;如果键已经存在,则不做任何操作并返回当前键对应的值。

语法
- public V putIfAbsent(K key, V value)
返回值
如果键不存在且成功插入,则返回null。
如果键已经存在,则返回当前键对应的值,不进行插入操作。
putIfAbsent的实现原理
putIfAbsent方法通过以下步骤实现:
1、检查键是否存在:首先调用get方法获取键对应的值。

2、判断值是否为null:如果值为null,表示键不存在。
3、插入键值对:如果键不存在,则调用put方法将键值对插入到Map中。
4、返回结果:根据上述操作的结果返回相应的值。
常见错误及解决方案
错误一:未正确处理返回值
在使用putIfAbsent时,常见的错误是没有正确处理返回值。
- Map<String, String> map = new ConcurrentHashMap<>();
- map.putIfAbsent("key", "value");
- System.out.println(map.get("key")); // 可能输出null
解决方案:需要检查putIfAbsent的返回值,并根据返回值决定后续操作。
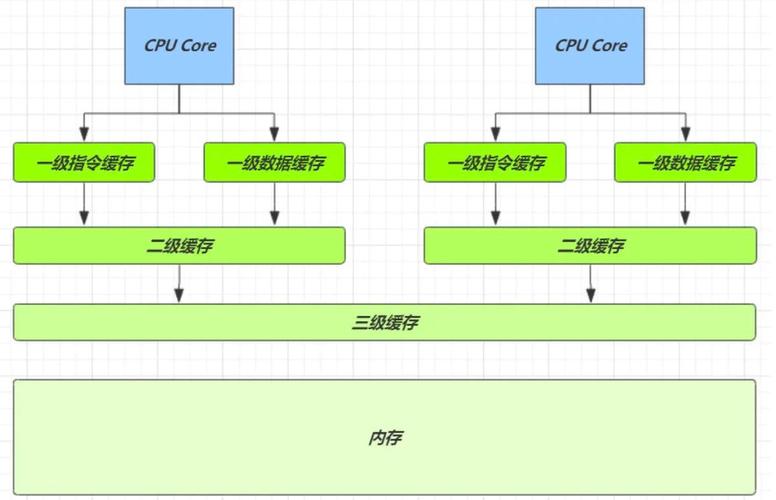
- String previousValue = map.putIfAbsent("key", "value");
- if (previousValue == null) {
- System.out.println("Key was not present, value added.");
- } else {
- System.out.println("Key already exists, current value: " + previousValue);
- }
错误二:误用put代替putIfAbsent
另一个常见的错误是在并发环境中误用put代替putIfAbsent,导致数据不一致。
- // 错误示例
- map.put("key", "newValue"); // 可能导致覆盖现有值
解决方案:在并发环境下,应使用putIfAbsent或其他线程安全的更新方法。
- String previousValue = map.putIfAbsent("key", "newValue");
- if (previousValue != null) {
- // 处理已有值的情况
- }
错误三:忽略返回值导致逻辑错误
有时开发者可能会忽略putIfAbsent的返回值,导致逻辑上的错误。
- // 错误示例
- map.putIfAbsent("key", "value");
- // 假设此处有其他线程修改了"key"的值
- boolean isPresent = map.containsKey("key");
- System.out.println(isPresent); // 可能输出false
解决方案:始终检查putIfAbsent的返回值,以确保逻辑的正确性。
- String previousValue = map.putIfAbsent("key", "value");
- if (previousValue == null) {
- // 确保"key"已被插入
- } else {
- // 处理已有值的情况
- }
代码示例
以下是一个完整的示例,展示了如何在并发环境中正确使用putIfAbsent方法:
- import java.util.concurrent.ConcurrentHashMap;
- import java.util.Map;
- public class PutIfAbsentExample {
- private final static Map<String, String> map = new ConcurrentHashMap<>();
- public static void main(String[] args) {
- // 线程1尝试添加键值对
- new Thread(() > {
- String previousValue = map.putIfAbsent("key1", "value1");
- if (previousValue == null) {
- System.out.println("Thread1: Key was not present, value added.");
- } else {
- System.out.println("Thread1: Key already exists, current value: " + previousValue);
- }
- }).start();
- // 线程2尝试添加相同的键值对
- new Thread(() > {
- String previousValue = map.putIfAbsent("key1", "value2");
- if (previousValue == null) {
- System.out.println("Thread2: Key was not present, value added.");
- } else {
- System.out.println("Thread2: Key already exists, current value: " + previousValue);
- }
- }).start();
- }
- }
输出可能为:
- Thread1: Key was not present, value added.
- Thread2: Key already exists, current value: value1
在这个示例中,线程1成功插入了键值对,而线程2检测到键已存在,因此没有覆盖原有值。
常见问题解答(FAQs)
Q1:putIfAbsent方法是否保证原子性?
A1:是的,putIfAbsent方法是原子性的,它确保在并发环境下,多个线程同时调用putIfAbsent时,只有一个线程能够成功插入键值对,其他线程将不会覆盖已有的值。
Q2:putIfAbsent与computeIfAbsent有什么区别?
A2:putIfAbsent仅在键不存在时插入值,并返回null或现有值,而computeIfAbsent会在键不存在时计算并插入值,无论键是否存在都会返回当前值,computeIfAbsent提供了更灵活的操作方式,可以根据键的存在与否执行不同的逻辑。
Q3:何时使用putIfAbsent?
A3:当需要在并发环境下安全地向Map中添加键值对,并且不希望覆盖已有的值时,可以使用putIfAbsent,在缓存系统中初始化默认值或在分布式系统中分配唯一标识符等场景下,putIfAbsent非常有用。
putIfAbsent是Java并发编程中的一个重要工具,用于在多线程环境下安全地向Map中添加键值对,正确理解和使用putIfAbsent可以避免常见的并发问题,提高程序的稳定性和可靠性,通过本文的介绍,希望读者能够更好地掌握putIfAbsent的使用方法及其注意事项。