PRopertyinfo报错
在使用.NET的反射机制时,PropertyInfo类是常用的工具之一,由于其灵活性和复杂性,开发者在使用PropertyInfo时常常会遇到各种错误,本文将详细探讨PropertyInfo报错的一些常见原因及其解决方法,并提供相关的示例代码和解释。
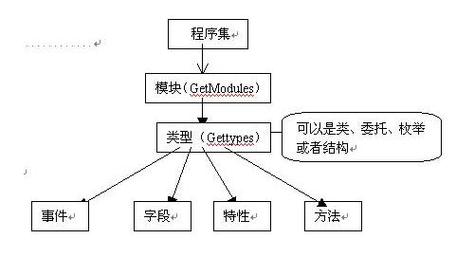
一、常见报错类型及解决方法
1. Type Mismatch Exception
描述:尝试将一个类型的值赋给另一个不兼容类型的属性时,会抛出TypeMismatchException。
示例:
public class Test { public string A { get; set; } } var test = new Test(); typeof(Test).GetField("A").SetValue(test, new Binding()); // 错误:不能将Binding类型赋值给string类型属性
解决方法:确保要设置的值的类型与属性的类型匹配,如果属性是字符串类型,则只能赋予字符串类型的值。
public class Test { public string A { get; set; } } var test = new Test(); typeof(Test).GetField("A").SetValue(test, "new value"); // 正确:赋值与属性类型匹配
2. ArgumentException
描述:传递错误的参数给方法时会引发ArgumentException。
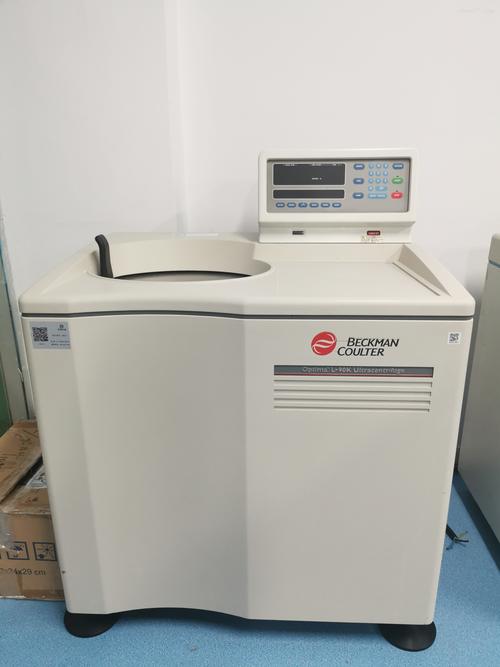
示例:
public class People { public string Name { get; set; } } delegate object MemberGetDelegate(People p); public class Program { public static void Main() { People people = new People { Name = "John" }; Type type = typeof(People); PropertyInfo property = type.GetProperty("Name"); MemberGetDelegate memberGet = (MemberGetDelegate)System.Delegate.CreateDelegate(typeof(MemberGetDelegate), property.GetGetMethod()); object value = memberGet(people); // 错误:类型不匹配 } }
解决方法:确保委托的返回类型与属性的返回类型一致。
public class People { public string Name { get; set; } } delegate string MemberGetDelegate(People p); // 修改委托的返回类型为string public class Program { public static void Main() { People people = new People { Name = "John" }; Type type = typeof(People); PropertyInfo property = type.GetProperty("Name"); System.Reflection.MethodInfo mi = property.GetGetMethod(); MemberGetDelegate memberGet = (MemberGetDelegate)System.Delegate.CreateDelegate(typeof(MemberGetDelegate), mi); object value = memberGet(people); // 正确:类型匹配 } }
3. AmbiguousMatchException
描述:当尝试获取属性或方法时,如果有多个重载且没有明确指定要使用哪个,则会引发AmbiguousMatchException。
示例:
public class Example { public void DoWork(int x) { } public void DoWork(string x) { } } Type exampleType = typeof(Example); PropertyInfo[] properties = exampleType.GetProperties(); // 可能引发AmbiguousMatchException
解决方法:明确指定要获取的属性或方法。
Type exampleType = typeof(Example); MethodInfo method = exampleType.GetMethod("DoWork", new Type[] { typeof(int) }); // 明确指定参数类型
4. NullReferenceException
描述:尝试访问或操作空对象时会引发NullReferenceException。
示例:
public class Example { public string Name { get; set; } } Example ex = null; PropertyInfo prop = ex.GetType().GetProperty("Name"); // 错误:ex为null,无法调用方法
解决方法:在使用对象之前检查是否为null。
if (ex != null) { PropertyInfo prop = ex.GetType().GetProperty("Name"); // 确保对象不为null }
二、PropertyInfo的使用注意事项
1、类型安全:始终确保在设置属性值时,值的类型与属性的类型匹配,否则会引发TypeMismatchException。
2、参数匹配:在使用反射创建委托时,确保委托的签名与目标方法的签名匹配,否则会引发ArgumentException。
3、明确指定:在获取属性或方法时,尽量明确指定名称和参数类型,以避免AmbiguousMatchException。
4、空值检查:在使用对象的方法或属性之前,始终检查对象是否为null,以避免NullReferenceException。
5、性能考虑:反射操作相对较慢,频繁使用时需考虑性能影响,可以使用缓存或其他优化手段提高性能。
6、安全性:反射可以绕过一些编译时的安全检查,因此在使用反射时要特别注意权限问题,避免潜在的安全风险。
7、文档和维护:由于反射代码通常不易读懂,建议在关键地方添加注释,并保持代码整洁,以便于维护。
8、测试覆盖:由于反射涉及动态行为,建议编写单元测试覆盖各种可能的情况,确保代码的健壮性。
9、异常处理:在使用反射时,应适当地捕获和处理异常,以防止程序崩溃,并提供有用的错误信息。
10、版本兼容性:注意反射在不同版本的.NET框架中的行为差异,确保代码在不同环境中都能正常运行。
三、FAQs
Q1:如何通过反射获取私有属性的值?
A1:可以通过BindingFlags.NonPublic来获取私有属性的值,下面是一个示例:
public class SecretHolder { private string Secret { get; set; } } SecretHolder holder = new SecretHolder { Secret = "TopSecret" }; PropertyInfo secretProp = typeof(SecretHolder).GetProperty("Secret", BindingFlags.NonPublic | BindingFlags.Instance); object secretValue = secretProp.GetValue(holder, null); // "TopSecret"
在这个示例中,我们使用了BindingFlags.NonPublic来指定我们要获取的是私有属性,然后通过GetValue方法获取属性的值,这种方法可以用于访问类的私有成员,但需要注意不要滥用此功能,以免破坏类的封装性。
Q2:如何判断某个属性是否为只读?
A2:可以通过检查属性的CanWrite属性来判断是否为只读,下面是一个示例:
public class ReadOnlyExample { public int ReadOnlyProperty { get; private set; } = 42; } PropertyInfo prop = typeof(ReadOnlyExample).GetProperty("ReadOnlyProperty"); bool isReadOnly = !prop.CanWrite; // true,因为该属性是只读的
在这个示例中,我们首先获取了ReadOnlyExample类的ReadOnlyProperty属性的信息,然后通过检查CanWrite属性来判断该属性是否为只读,如果CanWrite为false,则表示属性是只读的;否则表示属性是可写的,这种方法可以帮助我们在运行时了解属性的可写性,从而做出相应的操作决策。